Check SWOT data in the cloud#
Jinbo Wang
Revision history
Created on - 04/03/2023 J.W.
Revised for SMCE platform - 04/28/2023 J.W.
System configuration#
Load the python libraries and setup the AWS S3FS object for reading data directly from cloud. Make sure you have the .netrc file in your root directory with your earthdata login account and password. You can follow these steps if you have not done so: https://podaac.github.io/tutorials/external/NASA_Earthdata_Authentication.html.
import pprint
import xarray as xr
import netCDF4 as nc4
import pylab as plt
from scipy import signal
import numpy as np
import swot_ssh_utils as swot
import hvplot.pandas # noqa
import hvplot.xarray # noqa
import cartopy.crs as ccrs
import holoviews as hv
import warnings
warnings.filterwarnings('ignore')
def init_S3FileSystem():
import requests
import s3fs
# Get authentication credentials from a JSON file
creds = requests.get('https://archive.swot.podaac.earthdata.nasa.gov/s3credentials').json()
# Initialize an S3FileSystem instance with the credentials
s3 = s3fs.S3FileSystem(anon=False,
key=creds['accessKeyId'],
secret=creds['secretAccessKey'],
token=creds['sessionToken'])
# Return the S3FileSystem instance
return s3
def plotit(ax,lon,lat,dat,vmin=-0.15,vmax=0.15):
proj = ccrs.PlateCarree()
extent = [lon.min(),lon.max(), lat.min(),lat.max()]
# Add the scatter plot
lon=lon.data.flatten()
lat=lat.data.flatten()
ax.scatter(lon, lat, c=dat, s=1, cmap=plt.cm.bwr,
transform=ccrs.PlateCarree(),
vmin=-0.15,vmax=0.15)
ax.coastlines(resolution='110m')
ax.gridlines(draw_labels=True)
ax.set_extent(extent, proj)
return
# Call the init_S3FileSystem function and assign the result to a variable named s3sys
s3sys = init_S3FileSystem()
lat_bounds=[34,37]
pass_number = '013' #California
#pass_number = '026' #California
pass_number='017';lat_bounds=[-6.5,-4.5]
#Med-sea
#pass_number='003';latmin=38.5;latmax=41.1
#pass_num='016';latmin=36.5;latmax=43.5
#pass_number='016';latmin=38.5;latmax=41.1
#lat_bounds=[latmin,latmax]
#thresshold_std=0.267
The following block searches for SWOT Expert data product and displays the names of the most recent four NetCDF files within PODAAC cloud.
The ss=’SWOT_L2_LR_SSH_1.0’ specify the product collection short_name.
“s3://podaac-swot-ops-cumulus-protected” is the podaac S3 bucket.
Basic product#
product_type='Basic'
ss='SWOT_L2_LR_SSH_1.0/'
fns=s3sys.glob(f"s3://podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/*{product_type}*_{pass_number}_*.nc")
print(f"There are {len(fns)} files in the system. The most recent four files are the following.")
pprint.pprint(fns[-4:])
There are 90 files in the system. The most recent four files are the following.
['podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/SWOT_L2_LR_SSH_Basic_544_017_20230607T004843_20230607T013451_PIA1_01.nc',
'podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/SWOT_L2_LR_SSH_Basic_545_017_20230608T003921_20230608T012529_PIA1_01.nc',
'podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/SWOT_L2_LR_SSH_Basic_546_017_20230609T002959_20230609T011607_PIA1_01.nc',
'podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/SWOT_L2_LR_SSH_Basic_547_017_20230610T002037_20230610T010645_PIA1_01.nc']
Use the python class SSH_L2 to access to the prebuilt functions.#
data=swot.SSH_L2()
data.load_data(fns[-1],lat_bounds=lat_bounds,s3sys=s3sys)
display(data.Basic)
<xarray.Dataset> Dimensions: (num_lines: 112, num_pixels: 69, num_sides: 2) Coordinates: latitude (num_lines, num_pixels) float64 -6... longitude (num_lines, num_pixels) float64 17... Dimensions without coordinates: num_lines, num_pixels, num_sides Data variables: (12/24) time (num_lines) datetime64[ns] 2023-06... time_tai (num_lines) datetime64[ns] 2023-06... ssh_karin (num_lines, num_pixels) float64 na... ssh_karin_qual (num_lines, num_pixels) float64 3.... ssh_karin_uncert (num_lines, num_pixels) float32 na... ssha_karin (num_lines, num_pixels) float64 na... ... ... mean_sea_surface_cnescls (num_lines, num_pixels) float64 34... mean_sea_surface_cnescls_uncert (num_lines, num_pixels) float32 0.... geoid (num_lines, num_pixels) float64 33... internal_tide_hret (num_lines, num_pixels) float32 -0... height_cor_xover (num_lines, num_pixels) float64 -0... height_cor_xover_qual (num_lines, num_pixels) float32 2.... Attributes: (12/60) Conventions: CF-1.7 title: Level 2 Low Rate Sea Surfa... source: Ka-band radar interferometer history: 2023-06-12T22:33:34Z : Cre... platform: SWOT reference_document: D-56407_SWOT_Product_Descr... ... ... ellipsoid_flattening: 0.0033528106647474805 institution: CNES references: V1.1 equator_time: 2023-06-10T00:41:13.758000Z equator_longitude: 176.73 product_version: 01
- num_lines: 112
- num_pixels: 69
- num_sides: 2
- latitude(num_lines, num_pixels)float64-6.413 -6.416 ... -4.605 -4.608
- long_name :
- latitude (positive N, negative S)
- standard_name :
- latitude
- units :
- degrees_north
- valid_min :
- -80000000
- valid_max :
- 80000000
- comment :
- Latitude of measurement [-80,80]. Positive latitude is North latitude, negative latitude is South latitude.
array([[-6.413136, -6.415818, -6.4185 , ..., -6.58883 , -6.591471, -6.594111], [-6.395255, -6.397938, -6.400619, ..., -6.570937, -6.573578, -6.576218], [-6.377375, -6.380057, -6.382738, ..., -6.553044, -6.555685, -6.558325], ..., [-4.463831, -4.466491, -4.469149, ..., -4.638398, -4.641028, -4.643657], [-4.445945, -4.448604, -4.451263, ..., -4.620503, -4.623133, -4.625762], [-4.428059, -4.430718, -4.433376, ..., -4.602608, -4.605238, -4.607867]])
- longitude(num_lines, num_pixels)float64175.2 175.2 175.2 ... 176.7 176.7
- long_name :
- longitude (degrees East)
- standard_name :
- longitude
- units :
- degrees_east
- valid_min :
- 0
- valid_max :
- 359999999
- comment :
- Longitude of measurement. East longitude relative to Greenwich meridian.
array([[175.173285, 175.191164, 175.209043, ..., 176.353686, 176.371577, 176.389468], [175.175968, 175.193847, 175.211725, ..., 176.356329, 176.37422 , 176.392111], [175.178651, 175.196529, 175.214407, ..., 176.358972, 176.376862, 176.394752], ..., [175.46394 , 175.481765, 175.49959 , ..., 176.640654, 176.658488, 176.676321], [175.466591, 175.484416, 175.50224 , ..., 176.643278, 176.661111, 176.678944], [175.469242, 175.487066, 175.50489 , ..., 176.645901, 176.663734, 176.681566]])
- time(num_lines)datetime64[ns]...
- long_name :
- time in UTC
- standard_name :
- time
- tai_utc_difference :
- 37.0
- leap_second :
- 0000-00-00T00:00:00Z
- comment :
- Time of measurement in seconds in the UTC time scale since 1 Jan 2000 00:00:00 UTC. [tai_utc_difference] is the difference between TAI and UTC reference time (seconds) for the first measurement of the data set. If a leap second occurs within the data set, the attribute leap_second is set to the UTC time at which the leap second occurs.
array(['2023-06-10T00:39:20.978667648', '2023-06-10T00:39:21.288906624', '2023-06-10T00:39:21.599270144', '2023-06-10T00:39:21.909661568', '2023-06-10T00:39:22.219882624', '2023-06-10T00:39:22.530306944', '2023-06-10T00:39:22.840509312', '2023-06-10T00:39:23.150892800', '2023-06-10T00:39:23.461174656', '2023-06-10T00:39:23.771452800', '2023-06-10T00:39:24.081837312', '2023-06-10T00:39:24.392035712', '2023-06-10T00:39:24.702454272', '2023-06-10T00:39:25.012669952', '2023-06-10T00:39:25.323063552', '2023-06-10T00:39:25.633432064', '2023-06-10T00:39:25.943667200', '2023-06-10T00:39:26.254076544', '2023-06-10T00:39:26.564272256', '2023-06-10T00:39:26.874670592', '2023-06-10T00:39:27.184928000', '2023-06-10T00:39:27.495228544', '2023-06-10T00:39:27.805590144', '2023-06-10T00:39:28.115796992', '2023-06-10T00:39:28.426213376', '2023-06-10T00:39:28.736412032', '2023-06-10T00:39:29.046871936', '2023-06-10T00:39:29.357245056', '2023-06-10T00:39:29.667483392', '2023-06-10T00:39:29.977885568', '2023-06-10T00:39:30.288078848', '2023-06-10T00:39:30.598485888', '2023-06-10T00:39:30.908721152', '2023-06-10T00:39:31.219046656', '2023-06-10T00:39:31.529382784', '2023-06-10T00:39:31.839605760', '2023-06-10T00:39:32.150011264', '2023-06-10T00:39:32.460202880', '2023-06-10T00:39:32.770748032', '2023-06-10T00:39:33.081122048', '2023-06-10T00:39:33.391369984', '2023-06-10T00:39:33.701757312', '2023-06-10T00:39:34.011946752', '2023-06-10T00:39:34.322358400', '2023-06-10T00:39:34.632571648', '2023-06-10T00:39:34.942918144', '2023-06-10T00:39:35.253225216', '2023-06-10T00:39:35.563470464', '2023-06-10T00:39:35.873856640', '2023-06-10T00:39:36.184058752', '2023-06-10T00:39:36.494707840', '2023-06-10T00:39:36.805083520', '2023-06-10T00:39:37.115336832', '2023-06-10T00:39:37.425709056', '2023-06-10T00:39:37.735902464', '2023-06-10T00:39:38.046314112', '2023-06-10T00:39:38.356511360', '2023-06-10T00:39:38.666872064', '2023-06-10T00:39:38.977157120', '2023-06-10T00:39:39.287418368', '2023-06-10T00:39:39.597790848', '2023-06-10T00:39:39.907995520', '2023-06-10T00:39:40.218567552', '2023-06-10T00:39:40.528857344', '2023-06-10T00:39:40.839147392', '2023-06-10T00:39:41.149487616', '2023-06-10T00:39:41.459690752', '2023-06-10T00:39:41.770093696', '2023-06-10T00:39:42.080275712', '2023-06-10T00:39:42.390657408', '2023-06-10T00:39:42.700903808', '2023-06-10T00:39:43.011191168', '2023-06-10T00:39:43.321530880', '2023-06-10T00:39:43.631768832', '2023-06-10T00:39:43.942450048', '2023-06-10T00:39:44.252738560', '2023-06-10T00:39:44.563035520', '2023-06-10T00:39:44.873359744', '2023-06-10T00:39:45.183569920', '2023-06-10T00:39:45.493962112', '2023-06-10T00:39:45.804136448', '2023-06-10T00:39:46.114519808', '2023-06-10T00:39:46.424747264', '2023-06-10T00:39:46.735046912', '2023-06-10T00:39:47.045373696', '2023-06-10T00:39:47.355605376', '2023-06-10T00:39:47.666151680', '2023-06-10T00:39:47.976369920', '2023-06-10T00:39:48.286706560', '2023-06-10T00:39:48.596991744', '2023-06-10T00:39:48.907226112', '2023-06-10T00:39:49.217602560', '2023-06-10T00:39:49.527771648', '2023-06-10T00:39:49.838161536', '2023-06-10T00:39:50.148363136', '2023-06-10T00:39:50.458678656', '2023-06-10T00:39:50.768977024', '2023-06-10T00:39:51.079220736', '2023-06-10T00:39:51.389733760', '2023-06-10T00:39:51.699925376', '2023-06-10T00:39:52.010282240', '2023-06-10T00:39:52.320537344', '2023-06-10T00:39:52.630795776', '2023-06-10T00:39:52.941152128', '2023-06-10T00:39:53.251321472', '2023-06-10T00:39:53.561717376', '2023-06-10T00:39:53.871900800', '2023-06-10T00:39:54.182224768', '2023-06-10T00:39:54.492516224', '2023-06-10T00:39:54.802716032', '2023-06-10T00:39:55.113065600', '2023-06-10T00:39:55.423226752'], dtype='datetime64[ns]')
- time_tai(num_lines)datetime64[ns]...
- long_name :
- time in TAI
- standard_name :
- time
- tai_utc_difference :
- 37.0
- comment :
- Time of measurement in seconds in the TAI time scale since 1 Jan 2000 00:00:00 TAI. This time scale contains no leap seconds. The difference (in seconds) with time in UTC is given by the attribute [time:tai_utc_difference].
array(['2023-06-10T00:39:57.978667520', '2023-06-10T00:39:58.288906752', '2023-06-10T00:39:58.599269760', '2023-06-10T00:39:58.909660928', '2023-06-10T00:39:59.219882880', '2023-06-10T00:39:59.530306816', '2023-06-10T00:39:59.840509312', '2023-06-10T00:40:00.150893440', '2023-06-10T00:40:00.461175424', '2023-06-10T00:40:00.771452544', '2023-06-10T00:40:01.081837568', '2023-06-10T00:40:01.392035584', '2023-06-10T00:40:01.702454400', '2023-06-10T00:40:02.012670080', '2023-06-10T00:40:02.323062784', '2023-06-10T00:40:02.633431424', '2023-06-10T00:40:02.943667712', '2023-06-10T00:40:03.254077056', '2023-06-10T00:40:03.564272000', '2023-06-10T00:40:03.874671488', '2023-06-10T00:40:04.184927872', '2023-06-10T00:40:04.495228544', '2023-06-10T00:40:04.805590528', '2023-06-10T00:40:05.115796992', '2023-06-10T00:40:05.426214016', '2023-06-10T00:40:05.736412544', '2023-06-10T00:40:06.046872576', '2023-06-10T00:40:06.357245056', '2023-06-10T00:40:06.667483648', '2023-06-10T00:40:06.977885952', '2023-06-10T00:40:07.288078208', '2023-06-10T00:40:07.598486016', '2023-06-10T00:40:07.908721024', '2023-06-10T00:40:08.219046656', '2023-06-10T00:40:08.529382272', '2023-06-10T00:40:08.839606144', '2023-06-10T00:40:09.150010752', '2023-06-10T00:40:09.460203136', '2023-06-10T00:40:09.770747008', '2023-06-10T00:40:10.081122048', '2023-06-10T00:40:10.391369472', '2023-06-10T00:40:10.701757568', '2023-06-10T00:40:11.011946880', '2023-06-10T00:40:11.322358656', '2023-06-10T00:40:11.632572160', '2023-06-10T00:40:11.942917504', '2023-06-10T00:40:12.253224960', '2023-06-10T00:40:12.563470336', '2023-06-10T00:40:12.873856384', '2023-06-10T00:40:13.184057984', '2023-06-10T00:40:13.494707712', '2023-06-10T00:40:13.805083008', '2023-06-10T00:40:14.115336320', '2023-06-10T00:40:14.425709312', '2023-06-10T00:40:14.735902080', '2023-06-10T00:40:15.046313728', '2023-06-10T00:40:15.356511872', '2023-06-10T00:40:15.666872192', '2023-06-10T00:40:15.977157376', '2023-06-10T00:40:16.287418496', '2023-06-10T00:40:16.597790848', '2023-06-10T00:40:16.907995648', '2023-06-10T00:40:17.218566784', '2023-06-10T00:40:17.528857984', '2023-06-10T00:40:17.839147392', '2023-06-10T00:40:18.149486976', '2023-06-10T00:40:18.459690624', '2023-06-10T00:40:18.770092800', '2023-06-10T00:40:19.080275328', '2023-06-10T00:40:19.390657920', '2023-06-10T00:40:19.700903552', '2023-06-10T00:40:20.011191296', '2023-06-10T00:40:20.321530240', '2023-06-10T00:40:20.631769600', '2023-06-10T00:40:20.942449920', '2023-06-10T00:40:21.252738176', '2023-06-10T00:40:21.563036032', '2023-06-10T00:40:21.873359744', '2023-06-10T00:40:22.183570560', '2023-06-10T00:40:22.493962112', '2023-06-10T00:40:22.804136576', '2023-06-10T00:40:23.114520448', '2023-06-10T00:40:23.424747776', '2023-06-10T00:40:23.735047168', '2023-06-10T00:40:24.045374080', '2023-06-10T00:40:24.355605376', '2023-06-10T00:40:24.666151680', '2023-06-10T00:40:24.976369152', '2023-06-10T00:40:25.286707072', '2023-06-10T00:40:25.596992256', '2023-06-10T00:40:25.907226112', '2023-06-10T00:40:26.217602944', '2023-06-10T00:40:26.527771648', '2023-06-10T00:40:26.838161536', '2023-06-10T00:40:27.148363008', '2023-06-10T00:40:27.458678784', '2023-06-10T00:40:27.768976768', '2023-06-10T00:40:28.079220864', '2023-06-10T00:40:28.389734016', '2023-06-10T00:40:28.699925376', '2023-06-10T00:40:29.010282112', '2023-06-10T00:40:29.320536960', '2023-06-10T00:40:29.630795392', '2023-06-10T00:40:29.941152640', '2023-06-10T00:40:30.251322368', '2023-06-10T00:40:30.561717376', '2023-06-10T00:40:30.871901568', '2023-06-10T00:40:31.182224896', '2023-06-10T00:40:31.492516224', '2023-06-10T00:40:31.802715904', '2023-06-10T00:40:32.113065472', '2023-06-10T00:40:32.423227136'], dtype='datetime64[ns]')
- ssh_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using radiometer measurements for wet troposphere effects on the KaRIn measurement (e.g., rad_wet_tropo_cor and sea_state_bias_cor).
array([[ nan, nan, 35.2186, ..., 31.695 , nan, nan], [ nan, nan, 35.1478, ..., 31.7385, nan, nan], [ nan, nan, 35.1011, ..., 31.8088, nan, nan], ..., [ nan, nan, 31.0551, ..., 29.5036, nan, nan], [ nan, nan, 31.0637, ..., 29.3946, nan, nan], [ nan, nan, 31.0827, ..., 29.2924, nan, nan]])
- ssh_karin_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4194811391
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_uncert(num_lines, num_pixels)float32...
- long_name :
- sea surface height anomaly uncertainty
- units :
- m
- valid_min :
- 0
- valid_max :
- 60000
- comment :
- 1-sigma uncertainty on the sea surface height from the KaRIn measurement.
array([[ nan, nan, 0.0077, ..., 0.0075, nan, nan], [ nan, nan, 0.0072, ..., 0.0081, nan, nan], [ nan, nan, 0.0071, ..., 0.0079, nan, nan], ..., [ nan, nan, 0.0073, ..., 0.0094, nan, nan], [ nan, nan, 0.0087, ..., 0.0083, nan, nan], [ nan, nan, 0.0083, ..., 0.0087, nan, nan]], dtype=float32)
- ssha_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.8231, ..., -0.9348, nan, nan], [ nan, nan, 0.8025, ..., -0.9491, nan, nan], [ nan, nan, 0.8071, ..., -0.943 , nan, nan], ..., [ nan, nan, 0.8175, ..., -0.7904, nan, nan], [ nan, nan, 0.8173, ..., -0.8014, nan, nan], [ nan, nan, 0.8217, ..., -0.8028, nan, nan]])
- ssha_karin_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4261920255
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_2_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using model-based estimates for wet troposphere effects on the KaRIn measurement (e.g., model_wet_tropo_cor and sea_state_bias_cor_2).
array([[ nan, nan, 35.222 , ..., 31.7584, nan, nan], [ nan, nan, 35.1506, ..., 31.8031, nan, nan], [ nan, nan, 35.1033, ..., 31.875 , nan, nan], ..., [ nan, nan, 31.1026, ..., 29.5253, nan, nan], [ nan, nan, 31.1108, ..., 29.4178, nan, nan], [ nan, nan, 31.13 , ..., 29.3168, nan, nan]])
- ssh_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3792158207
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin_2 variable.
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- ssha_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_2_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin_2 - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.8265, ..., -0.8714, nan, nan], [ nan, nan, 0.8053, ..., -0.8845, nan, nan], [ nan, nan, 0.8093, ..., -0.8768, nan, nan], ..., [ nan, nan, 0.865 , ..., -0.7687, nan, nan], [ nan, nan, 0.8644, ..., -0.7782, nan, nan], [ nan, nan, 0.869 , ..., -0.7784, nan, nan]])
- ssha_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3859267071
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin_2 variable
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- num_pt_avg(num_lines, num_pixels)float32...
- long_name :
- number of samples averaged
- units :
- 1
- valid_min :
- 0
- valid_max :
- 289
- comment :
- Number of native unsmoothed, beam-combined KaRIn samples averaged.
array([[ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], ..., [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.]], dtype=float32)
- distance_to_coast(num_lines, num_pixels)float32...
- long_name :
- distance to coast
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- units :
- m
- valid_min :
- 0
- valid_max :
- 21000
- comment :
- Approximate distance to the nearest coast point along the Earth surface.
array([[130000., 128000., 124000., ..., 30000., 30000., 32000.], [128000., 126000., 124000., ..., 28000., 28000., 30000.], [128000., 126000., 124000., ..., 26000., 26000., 27000.], ..., [147000., 146000., 145000., ..., 126000., 128000., 127000.], [148000., 148000., 147000., ..., 128000., 129000., 128000.], [150000., 149000., 148000., ..., 130000., 131000., 132000.]], dtype=float32)
- heading_to_coast(num_lines, num_pixels)float32...
- long_name :
- heading to coast
- units :
- degrees
- valid_min :
- 0
- valid_max :
- 35999
- comment :
- Approximate compass heading (0-360 degrees with respect to true north) to the nearest coast point.
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], dtype=float32)
- ancillary_surface_classification_flag(num_lines, num_pixels)float32...
- long_name :
- surface classification
- standard_name :
- status_flag
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- flag_meanings :
- open_ocean land continental_water aquatic_vegetation continental_ice_snow floating_ice salted_basin
- flag_values :
- [0 1 2 3 4 5 6]
- valid_min :
- 0
- valid_max :
- 6
- comment :
- 7-state surface type classification computed from a mask built with MODIS and GlobCover data.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- dynamic_ice_flag(num_lines, num_pixels)float32...
- long_name :
- dynamic ice flag
- standard_name :
- status_flag
- source :
- EUMETSAT Ocean and Sea Ice Satellite Applications Facility
- institution :
- EUMETSAT
- flag_meanings :
- no_ice probable_ice ice no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Dynamic ice flag for the location of the KaRIn measurement.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rain_flag(num_lines, num_pixels)float32...
- long_name :
- rain flag
- standard_name :
- status_flag
- flag_meanings :
- no_rain probable_rain rain no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Flag indicates that signal is attenuated, probably from rain.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rad_surface_type_flag(num_lines, num_sides)float32...
- long_name :
- radiometer surface type flag
- standard_name :
- status_flag
- source :
- Advanced Microwave Radiometer
- flag_meanings :
- open_ocean coastal_ocean land
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the validity and type of processing applied to generate the wet troposphere correction (rad_wet_tropo_cor). A value of 0 indicates that open ocean processing is used, a value of 1 indicates coastal processing, and a value of 2 indicates that rad_wet_tropo_cor is invalid due to land contamination.
array([[1., 0.], [1., 0.], [1., 0.], ..., [0., 0.], [0., 0.], [0., 0.]], dtype=float32)
- mean_sea_surface_cnescls(num_lines, num_pixels)float64...
- long_name :
- mean sea surface height (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Mean sea surface height above the reference ellipsoid. The value is referenced to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[34.3678, 34.335 , 34.306 , ..., 32.5267, 32.501 , 32.4758], [34.3202, 34.2876, 34.2562, ..., 32.5841, 32.5518, 32.5209], [34.2707, 34.2378, 34.2054, ..., 32.6479, 32.612 , 32.5783], ..., [30.1714, 30.1627, 30.1533, ..., 30.2082, 30.0953, 29.9772], [30.1742, 30.1672, 30.1616, ..., 30.1103, 30.0095, 29.9012], [30.1834, 30.1789, 30.1758, ..., 30.0096, 29.919 , 29.8205]])
- mean_sea_surface_cnescls_uncert(num_lines, num_pixels)float32...
- long_name :
- mean sea surface height accuracy (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- 0
- valid_max :
- 10000
- comment :
- Accuracy of the mean sea surface height (mean_sea_surface_cnescls).
array([[0.0168, 0.0166, 0.0163, ..., 0.0175, 0.0172, 0.0173], [0.017 , 0.0167, 0.0165, ..., 0.0172, 0.0175, 0.0177], [0.0171, 0.017 , 0.0167, ..., 0.0171, 0.0175, 0.0175], ..., [0.0163, 0.0166, 0.0159, ..., 0.0153, 0.0174, 0.0151], [0.0166, 0.0168, 0.016 , ..., 0.0142, 0.0155, 0.0123], [0.0166, 0.0167, 0.016 , ..., 0.0124, 0.0129, 0.0082]], dtype=float32)
- geoid(num_lines, num_pixels)float64...
- long_name :
- geoid height
- standard_name :
- geoid_height_above_reference_ellipsoid
- source :
- EGM2008 (Pavlis et al., 2012)
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Geoid height above the reference ellipsoid with a correction to refer the value to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[33.4881, 33.45 , 33.4112, ..., 31.8215, 31.8038, 31.7851], [33.4404, 33.4013, 33.3618, ..., 31.8821, 31.8611, 31.8388], [33.3917, 33.3519, 33.3116, ..., 31.9504, 31.9256, 31.899 ], ..., [29.3551, 29.3505, 29.3443, ..., 29.4515, 29.3589, 29.2499], [29.3622, 29.359 , 29.3541, ..., 29.3487, 29.2626, 29.1624], [29.3732, 29.3718, 29.3688, ..., 29.2393, 29.1606, 29.0699]])
- internal_tide_hret(num_lines, num_pixels)float32...
- long_name :
- coherent internal tide (HRET)
- source :
- Zaron (2019)
- units :
- m
- valid_min :
- -2000
- valid_max :
- 2000
- comment :
- Coherent internal ocean tide. This value is subtracted from the ssh_karin and ssh_karin_2 to compute ssha_karin and ssha_karin_2, respectively.
array([[-0.0077, -0.0077, -0.0077, ..., -0.0012, -0.0002, 0.0008], [-0.008 , -0.008 , -0.008 , ..., -0.0006, 0.0004, 0.0014], [-0.0082, -0.0082, -0.0083, ..., 0. , 0.001 , 0.002 ], ..., [ 0.0061, 0.0063, 0.0066, ..., 0.0015, 0.0012, 0.0008], [ 0.0069, 0.0071, 0.0073, ..., 0.0016, 0.0013, 0.0009], [ 0.0075, 0.0077, 0.0079, ..., 0.0017, 0.0014, 0.001 ]], dtype=float32)
- height_cor_xover(num_lines, num_pixels)float64...
- long_name :
- height correction from crossover calibration
- units :
- m
- quality_flag :
- height_cor_xover_qual
- valid_min :
- -100000
- valid_max :
- 100000
- comment :
- Height correction from crossover calibration. To apply this correction the value of height_cor_xover should be added to the value of ssh_karin, ssh_karin_2, ssha_karin, and ssha_karin_2.
array([[-0.6912, -0.6778, -0.664 , ..., 0.9446, 0.9811, 1.018 ], [-0.6913, -0.6779, -0.6641, ..., 0.9447, 0.9812, 1.0181], [-0.6914, -0.678 , -0.6641, ..., 0.9448, 0.9813, 1.0182], ..., [-0.7015, -0.6878, -0.6736, ..., 0.9561, 0.9929, 1.0301], [-0.7016, -0.6879, -0.6737, ..., 0.9562, 0.993 , 1.0302], [-0.7017, -0.688 , -0.6738, ..., 0.9563, 0.9931, 1.0303]])
- height_cor_xover_qual(num_lines, num_pixels)float32...
- long_name :
- quality flag for height correction from crossover calibration
- standard_name :
- status_flag
- flag_meanings :
- good suspect bad
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the quality of the height correction from crossover calibration. Values of 0, 1, and 2 indicate that the correction is good, suspect, and bad, respectively.
array([[2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], ..., [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.]], dtype=float32)
- Conventions :
- CF-1.7
- title :
- Level 2 Low Rate Sea Surface Height Data Product - Basic SSH
- source :
- Ka-band radar interferometer
- history :
- 2023-06-12T22:33:34Z : Creation
- platform :
- SWOT
- reference_document :
- D-56407_SWOT_Product_Description_L2_LR_SSH
- contact :
- podaac@jpl.nasa.gov
- cycle_number :
- 547
- pass_number :
- 17
- short_name :
- L2_LR_SSH
- product_file_id :
- Basic
- crid :
- PIA1
- pge_name :
- PGE_L2_LR_SSH
- pge_version :
- 4.3.0
- time_coverage_start :
- 2023-06-10T00:20:37.128115
- time_coverage_end :
- 2023-06-10T01:06:45.350009
- geospatial_lon_min :
- 93.01029299999999
- geospatial_lon_max :
- 260.464899
- geospatial_lat_min :
- -78.272196
- geospatial_lat_max :
- 78.27232099999999
- left_first_longitude :
- 93.025939
- left_first_latitude :
- -77.053978
- left_last_longitude :
- 260.464899
- left_last_latitude :
- 78.272284
- right_first_longitude :
- 93.01029299999999
- right_first_latitude :
- -78.272148
- right_last_longitude :
- 260.45079599999997
- right_last_latitude :
- 77.054112
- wavelength :
- 0.008385803020979021
- transmit_antenna :
- minus_y
- xref_l1b_lr_intf_file :
- SWOT_L1B_LR_INTF_547_017_20230610T001535_20230610T010648_PIA1_01.nc
- xref_l2_nalt_gdr_files :
- SWOT_IPN_2PfP547_016_20230609_232433_20230610_001538.nc, SWOT_IPN_2PfP547_017_20230610_001538_20230610_010644.nc, SWOT_IPN_2PfP547_018_20230610_010644_20230610_015750.nc
- xref_l2_rad_gdr_files :
- SWOT_IPRAD_2PaP547_016_20230609_232429_20230610_001542_PIA1_01.nc, SWOT_IPRAD_2PaP547_017_20230610_001535_20230610_010648_PIA1_01.nc, SWOT_IPRAD_2PaP547_018_20230610_010640_20230610_015753_PIA1_01.nc
- xref_int_lr_xover_cal_file :
- SWOT_INT_LR_XOverCal_20230609T232423_20230610T231521_PIA1_01.nc
- xref_statickarincal_files :
- SWOT_StaticKaRInCalAdjustableParam_20000101T000000_20991231T235959_20230424T230000_v104.nc
- xref_param_l2_lr_precalssh_file :
- SWOT_Param_L2_LR_PreCalSSH_20000101T000000_20991231T235959_20230215T234922_v201.nc
- xref_orbit_ephemeris_file :
- SWOT_POR_AXVCNE20230611_105049_20230609_225923_20230611_005923.nc
- xref_reforbittrack_files :
- SWOT_RefOrbitTrack125mPass1_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt, SWOT_RefOrbitTrack125mPass2_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt
- xref_meteorological_sealevel_pressure_files :
- SMM_PMA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_PMA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_wettroposphere_files :
- SMM_WEA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_WEA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_wind_files :
- SMM_UWA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_VWA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_UWA_AXVCNE20230610_144913_20230610_060000_20230610_060000, SMM_VWA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_surface_pressure_files :
- SMM_PSA_AXVCNE20230610_054037_20230610_000000_20230610_000000, SMM_PSA_AXVCNE20230610_174038_20230610_060000_20230610_060000
- xref_meteorological_temperature_files :
- SMM_T2M_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_T2M_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_meteorological_water_vapor_files :
- SMM_CWV_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_CWV_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_meteorological_cloud_liquid_water_files :
- SMM_CLW_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_CLW_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_model_significant_wave_height_files :
- SMM_SWH_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_SWH_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_gim_files :
- JPLQ1610.23I
- xref_pole_location_file :
- SMM_PO1_AXXCNE20230612_200000_19900101_000000_20231210_000000
- xref_dac_files :
- SMM_MOG_AXPCNE20230610_074716_20230610_000000_20230610_000000, SMM_MOG_AXPCNE20230610_204501_20230610_060000_20230610_060000
- xref_precipitation_files :
- SMM_CRR_AXFCNE20230610_065537_20230610_000000_20230610_000000.grb, SMM_LSR_AXFCNE20230610_065537_20230610_000000_20230610_000000.grb, SMM_CRR_AXFCNE20230610_065537_20230610_060000_20230610_060000.grb, SMM_LSR_AXFCNE20230610_065537_20230610_060000_20230610_060000.grb
- xref_sea_ice_mask_files :
- SMM_ICS_AXFCNE20230611_042010_20230610_000000_20230610_235959.nc, SMM_ICN_AXFCNE20230611_041532_20230610_000000_20230610_235959.nc
- xref_wave_model_files :
- SMM_WMA_AXPCNE20230610_072015_20230609_030000_20230610_000000.grb, SMM_WMA_AXPCNE20230611_072014_20230610_030000_20230611_000000.grb
- xref_geco_database_version :
- v100
- ellipsoid_semi_major_axis :
- 6378137.0
- ellipsoid_flattening :
- 0.0033528106647474805
- institution :
- CNES
- references :
- V1.1
- equator_time :
- 2023-06-10T00:41:13.758000Z
- equator_longitude :
- 176.73
- product_version :
- 01
Plot the most recent 20 snapshots#
ni,nj=2,2
fig,ax=plt.subplots(ni,nj,figsize=(14,10),
subplot_kw={'projection': ccrs.PlateCarree()},
sharex=True,sharey=True)
axx=ax.flatten()
data=swot.SSH_L2()
for fn,ax0 in zip(fns[-ni*nj:],axx):
data.load_data(fn,lat_bounds=lat_bounds,s3sys=s3sys)
d=getattr(data,product_type)
lon,lat=d['longitude'][:],d['latitude'][:]
#The MSS in the product uses MSS15, which misses a lot of small-scale geoid. Replace it with MSS22 here:
da=d['ssha_karin_2'][:]+d['mean_sea_surface_cnescls']-swot.get_mss22(lon.data, lat.data)
da=da-np.nanmean(da,axis=0).reshape(1,-1)
#plotit(ax0,lon,lat,da)
display(d)
#da[d['ssha_karin_2_qual'].data>0]=np.nan
ax0.pcolor(lon,lat,da,vmin=-0.2,vmax=0.2)
ax0.set_title(fn.split('_')[-3][4:15])
plt.tight_layout()
<xarray.Dataset> Dimensions: (num_lines: 112, num_pixels: 69, num_sides: 2) Coordinates: latitude (num_lines, num_pixels) float64 -6... longitude (num_lines, num_pixels) float64 17... Dimensions without coordinates: num_lines, num_pixels, num_sides Data variables: (12/24) time (num_lines) datetime64[ns] 2023-06... time_tai (num_lines) datetime64[ns] 2023-06... ssh_karin (num_lines, num_pixels) float64 na... ssh_karin_qual (num_lines, num_pixels) float64 3.... ssh_karin_uncert (num_lines, num_pixels) float32 na... ssha_karin (num_lines, num_pixels) float64 na... ... ... mean_sea_surface_cnescls (num_lines, num_pixels) float64 34... mean_sea_surface_cnescls_uncert (num_lines, num_pixels) float32 0.... geoid (num_lines, num_pixels) float64 33... internal_tide_hret (num_lines, num_pixels) float32 0.... height_cor_xover (num_lines, num_pixels) float64 -0... height_cor_xover_qual (num_lines, num_pixels) float32 2.... Attributes: (12/60) Conventions: CF-1.7 title: Level 2 Low Rate Sea Surfa... source: Ka-band radar interferometer history: 2023-06-09T22:22:51Z : Cre... platform: SWOT reference_document: D-56407_SWOT_Product_Descr... ... ... ellipsoid_flattening: 0.0033528106647474805 institution: CNES references: V1.1 equator_time: 2023-06-07T01:09:20.307000Z equator_longitude: 176.73 product_version: 01
- num_lines: 112
- num_pixels: 69
- num_sides: 2
- latitude(num_lines, num_pixels)float64-6.413 -6.416 ... -4.605 -4.608
- long_name :
- latitude (positive N, negative S)
- standard_name :
- latitude
- units :
- degrees_north
- valid_min :
- -80000000
- valid_max :
- 80000000
- comment :
- Latitude of measurement [-80,80]. Positive latitude is North latitude, negative latitude is South latitude.
array([[-6.413136, -6.415818, -6.4185 , ..., -6.58883 , -6.591471, -6.594111], [-6.395255, -6.397938, -6.400619, ..., -6.570937, -6.573578, -6.576218], [-6.377375, -6.380057, -6.382738, ..., -6.553044, -6.555685, -6.558325], ..., [-4.463831, -4.466491, -4.469149, ..., -4.638398, -4.641028, -4.643657], [-4.445945, -4.448604, -4.451263, ..., -4.620503, -4.623133, -4.625762], [-4.428059, -4.430718, -4.433376, ..., -4.602608, -4.605238, -4.607867]])
- longitude(num_lines, num_pixels)float64175.2 175.2 175.2 ... 176.7 176.7
- long_name :
- longitude (degrees East)
- standard_name :
- longitude
- units :
- degrees_east
- valid_min :
- 0
- valid_max :
- 359999999
- comment :
- Longitude of measurement. East longitude relative to Greenwich meridian.
array([[175.173285, 175.191164, 175.209043, ..., 176.353686, 176.371577, 176.389468], [175.175968, 175.193847, 175.211725, ..., 176.356329, 176.37422 , 176.392111], [175.178651, 175.196529, 175.214407, ..., 176.358972, 176.376862, 176.394752], ..., [175.46394 , 175.481765, 175.49959 , ..., 176.640654, 176.658488, 176.676321], [175.466591, 175.484416, 175.50224 , ..., 176.643278, 176.661111, 176.678944], [175.469242, 175.487066, 175.50489 , ..., 176.645901, 176.663734, 176.681566]])
- time(num_lines)datetime64[ns]...
- long_name :
- time in UTC
- standard_name :
- time
- tai_utc_difference :
- 37.0
- leap_second :
- 0000-00-00T00:00:00Z
- comment :
- Time of measurement in seconds in the UTC time scale since 1 Jan 2000 00:00:00 UTC. [tai_utc_difference] is the difference between TAI and UTC reference time (seconds) for the first measurement of the data set. If a leap second occurs within the data set, the attribute leap_second is set to the UTC time at which the leap second occurs.
array(['2023-06-07T01:07:27.527832192', '2023-06-07T01:07:27.838214784', '2023-06-07T01:07:28.148508800', '2023-06-07T01:07:28.458767232', '2023-06-07T01:07:28.769181056', '2023-06-07T01:07:29.079333760', '2023-06-07T01:07:29.389500160', '2023-06-07T01:07:29.699634688', '2023-06-07T01:07:30.010015872', '2023-06-07T01:07:30.320326272', '2023-06-07T01:07:30.630570368', '2023-06-07T01:07:30.940982016', '2023-06-07T01:07:31.251175296', '2023-06-07T01:07:31.561571840', '2023-06-07T01:07:31.871836800', '2023-06-07T01:07:32.182116352', '2023-06-07T01:07:32.492514816', '2023-06-07T01:07:32.802651904', '2023-06-07T01:07:33.112809344', '2023-06-07T01:07:33.422944640', '2023-06-07T01:07:33.733340160', '2023-06-07T01:07:34.043622912', '2023-06-07T01:07:34.353884032', '2023-06-07T01:07:34.664281088', '2023-06-07T01:07:34.974472704', '2023-06-07T01:07:35.284880128', '2023-06-07T01:07:35.595122176', '2023-06-07T01:07:35.905422464', '2023-06-07T01:07:36.215803136', '2023-06-07T01:07:36.525922944', '2023-06-07T01:07:36.836054144', '2023-06-07T01:07:37.146190976', '2023-06-07T01:07:37.456600960', '2023-06-07T01:07:37.766853632', '2023-06-07T01:07:38.077136768', '2023-06-07T01:07:38.387515008', '2023-06-07T01:07:38.697706240', '2023-06-07T01:07:39.008123264', '2023-06-07T01:07:39.318342272', '2023-06-07T01:07:39.628680192', '2023-06-07T01:07:39.939009024', '2023-06-07T01:07:40.249202432', '2023-06-07T01:07:40.559514624', '2023-06-07T01:07:40.869689984', '2023-06-07T01:07:41.180090368', '2023-06-07T01:07:41.490331136', '2023-06-07T01:07:41.800628736', '2023-06-07T01:07:42.110985344', '2023-06-07T01:07:42.421182720', '2023-06-07T01:07:42.731598208', '2023-06-07T01:07:43.041796992', '2023-06-07T01:07:43.352155136', '2023-06-07T01:07:43.662451840', '2023-06-07T01:07:43.972690944', '2023-06-07T01:07:44.283062912', '2023-06-07T01:07:44.593246464', '2023-06-07T01:07:44.903651840', '2023-06-07T01:07:45.213875584', '2023-06-07T01:07:45.524193152', '2023-06-07T01:07:45.834525696', '2023-06-07T01:07:46.144735232', '2023-06-07T01:07:46.455142272', '2023-06-07T01:07:46.765325952', '2023-06-07T01:07:47.075705728', '2023-06-07T01:07:47.385961472', '2023-06-07T01:07:47.696272384', '2023-06-07T01:07:48.006705920', '2023-06-07T01:07:48.316893824', '2023-06-07T01:07:48.627301504', '2023-06-07T01:07:48.937509120', '2023-06-07T01:07:49.247845376', '2023-06-07T01:07:49.558152832', '2023-06-07T01:07:49.868381440', '2023-06-07T01:07:50.178772096', '2023-06-07T01:07:50.488950656', '2023-06-07T01:07:50.799350528', '2023-06-07T01:07:51.109549184', '2023-06-07T01:07:51.420053376', '2023-06-07T01:07:51.730666496', '2023-06-07T01:07:52.040846848', '2023-06-07T01:07:52.351251328', '2023-06-07T01:07:52.661452928', '2023-06-07T01:07:52.971801344', '2023-06-07T01:07:53.282088576', '2023-06-07T01:07:53.592333568', '2023-06-07T01:07:53.902714880', '2023-06-07T01:07:54.212893184', '2023-06-07T01:07:54.523292032', '2023-06-07T01:07:54.833498112', '2023-06-07T01:07:55.143853056', '2023-06-07T01:07:55.454217216', '2023-06-07T01:07:55.764421248', '2023-06-07T01:07:56.074825728', '2023-06-07T01:07:56.385005952', '2023-06-07T01:07:56.695382272', '2023-06-07T01:07:57.005634176', '2023-06-07T01:07:57.315907968', '2023-06-07T01:07:57.626267008', '2023-06-07T01:07:57.936452480', '2023-06-07T01:07:58.246856448', '2023-06-07T01:07:58.557038464', '2023-06-07T01:07:58.867507456', '2023-06-07T01:07:59.177915392', '2023-06-07T01:07:59.488119168', '2023-06-07T01:07:59.798511744', '2023-06-07T01:08:00.108683904', '2023-06-07T01:08:00.419067648', '2023-06-07T01:08:00.729291008', '2023-06-07T01:08:01.039592064', '2023-06-07T01:08:01.349914368', '2023-06-07T01:08:01.660124416', '2023-06-07T01:08:01.970514560'], dtype='datetime64[ns]')
- time_tai(num_lines)datetime64[ns]...
- long_name :
- time in TAI
- standard_name :
- time
- tai_utc_difference :
- 37.0
- comment :
- Time of measurement in seconds in the TAI time scale since 1 Jan 2000 00:00:00 TAI. This time scale contains no leap seconds. The difference (in seconds) with time in UTC is given by the attribute [time:tai_utc_difference].
array(['2023-06-07T01:08:04.527832064', '2023-06-07T01:08:04.838215296', '2023-06-07T01:08:05.148508672', '2023-06-07T01:08:05.458767488', '2023-06-07T01:08:05.769181312', '2023-06-07T01:08:06.079334144', '2023-06-07T01:08:06.389500544', '2023-06-07T01:08:06.699634816', '2023-06-07T01:08:07.010015872', '2023-06-07T01:08:07.320326400', '2023-06-07T01:08:07.630570240', '2023-06-07T01:08:07.940981760', '2023-06-07T01:08:08.251174784', '2023-06-07T01:08:08.561571456', '2023-06-07T01:08:08.871836928', '2023-06-07T01:08:09.182115968', '2023-06-07T01:08:09.492514048', '2023-06-07T01:08:09.802652160', '2023-06-07T01:08:10.112809600', '2023-06-07T01:08:10.422944256', '2023-06-07T01:08:10.733340160', '2023-06-07T01:08:11.043623168', '2023-06-07T01:08:11.353883904', '2023-06-07T01:08:11.664280832', '2023-06-07T01:08:11.974472576', '2023-06-07T01:08:12.284879872', '2023-06-07T01:08:12.595122304', '2023-06-07T01:08:12.905422336', '2023-06-07T01:08:13.215803136', '2023-06-07T01:08:13.525922944', '2023-06-07T01:08:13.836054144', '2023-06-07T01:08:14.146190336', '2023-06-07T01:08:14.456599936', '2023-06-07T01:08:14.766853888', '2023-06-07T01:08:15.077137408', '2023-06-07T01:08:15.387515008', '2023-06-07T01:08:15.697706880', '2023-06-07T01:08:16.008123136', '2023-06-07T01:08:16.318342784', '2023-06-07T01:08:16.628679424', '2023-06-07T01:08:16.939009408', '2023-06-07T01:08:17.249202304', '2023-06-07T01:08:17.559514112', '2023-06-07T01:08:17.869690112', '2023-06-07T01:08:18.180090752', '2023-06-07T01:08:18.490331648', '2023-06-07T01:08:18.800628480', '2023-06-07T01:08:19.110985600', '2023-06-07T01:08:19.421182720', '2023-06-07T01:08:19.731598080', '2023-06-07T01:08:20.041797504', '2023-06-07T01:08:20.352155264', '2023-06-07T01:08:20.662451840', '2023-06-07T01:08:20.972690688', '2023-06-07T01:08:21.283063040', '2023-06-07T01:08:21.593246720', '2023-06-07T01:08:21.903652352', '2023-06-07T01:08:22.213875584', '2023-06-07T01:08:22.524193024', '2023-06-07T01:08:22.834525184', '2023-06-07T01:08:23.144735104', '2023-06-07T01:08:23.455141760', '2023-06-07T01:08:23.765325696', '2023-06-07T01:08:24.075705984', '2023-06-07T01:08:24.385961472', '2023-06-07T01:08:24.696273024', '2023-06-07T01:08:25.006705280', '2023-06-07T01:08:25.316893184', '2023-06-07T01:08:25.627301120', '2023-06-07T01:08:25.937509376', '2023-06-07T01:08:26.247845760', '2023-06-07T01:08:26.558152320', '2023-06-07T01:08:26.868381056', '2023-06-07T01:08:27.178772224', '2023-06-07T01:08:27.488951808', '2023-06-07T01:08:27.799351296', '2023-06-07T01:08:28.109548800', '2023-06-07T01:08:28.420053120', '2023-06-07T01:08:28.730666752', '2023-06-07T01:08:29.040846464', '2023-06-07T01:08:29.351251072', '2023-06-07T01:08:29.661453440', '2023-06-07T01:08:29.971801088', '2023-06-07T01:08:30.282089088', '2023-06-07T01:08:30.592333568', '2023-06-07T01:08:30.902714880', '2023-06-07T01:08:31.212893696', '2023-06-07T01:08:31.523291264', '2023-06-07T01:08:31.833498624', '2023-06-07T01:08:32.143852800', '2023-06-07T01:08:32.454217216', '2023-06-07T01:08:32.764420864', '2023-06-07T01:08:33.074826112', '2023-06-07T01:08:33.385005696', '2023-06-07T01:08:33.695381632', '2023-06-07T01:08:34.005634688', '2023-06-07T01:08:34.315908224', '2023-06-07T01:08:34.626266496', '2023-06-07T01:08:34.936452096', '2023-06-07T01:08:35.246855936', '2023-06-07T01:08:35.557038592', '2023-06-07T01:08:35.867506688', '2023-06-07T01:08:36.177915136', '2023-06-07T01:08:36.488118912', '2023-06-07T01:08:36.798510976', '2023-06-07T01:08:37.108683904', '2023-06-07T01:08:37.419068160', '2023-06-07T01:08:37.729290880', '2023-06-07T01:08:38.039592064', '2023-06-07T01:08:38.349914368', '2023-06-07T01:08:38.660124032', '2023-06-07T01:08:38.970514944'], dtype='datetime64[ns]')
- ssh_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using radiometer measurements for wet troposphere effects on the KaRIn measurement (e.g., rad_wet_tropo_cor and sea_state_bias_cor).
array([[ nan, nan, 34.3935, ..., 30.9706, nan, nan], [ nan, nan, 34.3503, ..., 31.0314, nan, nan], [ nan, nan, 34.2964, ..., 31.1162, nan, nan], ..., [ nan, nan, 30.2887, ..., 28.8022, nan, nan], [ nan, nan, 30.3018, ..., 28.7078, nan, nan], [ nan, nan, 30.3373, ..., 28.6144, nan, nan]])
- ssh_karin_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4194811391
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_uncert(num_lines, num_pixels)float32...
- long_name :
- sea surface height anomaly uncertainty
- units :
- m
- valid_min :
- 0
- valid_max :
- 60000
- comment :
- 1-sigma uncertainty on the sea surface height from the KaRIn measurement.
array([[ nan, nan, 0.007 , ..., 0.0076, nan, nan], [ nan, nan, 0.0064, ..., 0.0077, nan, nan], [ nan, nan, 0.0074, ..., 0.0071, nan, nan], ..., [ nan, nan, 0.0075, ..., 0.0082, nan, nan], [ nan, nan, 0.0078, ..., 0.0088, nan, nan], [ nan, nan, 0.007 , ..., 0.0092, nan, nan]], dtype=float32)
- ssha_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.751 , ..., -0.9005, nan, nan], [ nan, nan, 0.7565, ..., -0.8981, nan, nan], [ nan, nan, 0.7526, ..., -0.8781, nan, nan], ..., [ nan, nan, 0.7789, ..., -0.7798, nan, nan], [ nan, nan, 0.7833, ..., -0.7768, nan, nan], [ nan, nan, 0.8041, ..., -0.7701, nan, nan]])
- ssha_karin_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4261920255
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_2_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using model-based estimates for wet troposphere effects on the KaRIn measurement (e.g., model_wet_tropo_cor and sea_state_bias_cor_2).
array([[ nan, nan, 34.4029, ..., 31.048 , nan, nan], [ nan, nan, 34.3601, ..., 31.1096, nan, nan], [ nan, nan, 34.3063, ..., 31.1968, nan, nan], ..., [ nan, nan, 30.3044, ..., 28.7999, nan, nan], [ nan, nan, 30.3174, ..., 28.7051, nan, nan], [ nan, nan, 30.3527, ..., 28.6114, nan, nan]])
- ssh_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3792158207
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin_2 variable.
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- ssha_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_2_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin_2 - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.7604, ..., -0.8231, nan, nan], [ nan, nan, 0.7663, ..., -0.8199, nan, nan], [ nan, nan, 0.7625, ..., -0.7975, nan, nan], ..., [ nan, nan, 0.7946, ..., -0.7821, nan, nan], [ nan, nan, 0.7989, ..., -0.7795, nan, nan], [ nan, nan, 0.8195, ..., -0.7731, nan, nan]])
- ssha_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3859267071
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin_2 variable
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- num_pt_avg(num_lines, num_pixels)float32...
- long_name :
- number of samples averaged
- units :
- 1
- valid_min :
- 0
- valid_max :
- 289
- comment :
- Number of native unsmoothed, beam-combined KaRIn samples averaged.
array([[ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], ..., [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.]], dtype=float32)
- distance_to_coast(num_lines, num_pixels)float32...
- long_name :
- distance to coast
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- units :
- m
- valid_min :
- 0
- valid_max :
- 21000
- comment :
- Approximate distance to the nearest coast point along the Earth surface.
array([[130000., 128000., 124000., ..., 30000., 30000., 32000.], [128000., 126000., 124000., ..., 28000., 28000., 30000.], [128000., 126000., 124000., ..., 26000., 26000., 27000.], ..., [147000., 146000., 145000., ..., 126000., 128000., 127000.], [148000., 148000., 147000., ..., 128000., 129000., 128000.], [150000., 149000., 148000., ..., 130000., 131000., 132000.]], dtype=float32)
- heading_to_coast(num_lines, num_pixels)float32...
- long_name :
- heading to coast
- units :
- degrees
- valid_min :
- 0
- valid_max :
- 35999
- comment :
- Approximate compass heading (0-360 degrees with respect to true north) to the nearest coast point.
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], dtype=float32)
- ancillary_surface_classification_flag(num_lines, num_pixels)float32...
- long_name :
- surface classification
- standard_name :
- status_flag
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- flag_meanings :
- open_ocean land continental_water aquatic_vegetation continental_ice_snow floating_ice salted_basin
- flag_values :
- [0 1 2 3 4 5 6]
- valid_min :
- 0
- valid_max :
- 6
- comment :
- 7-state surface type classification computed from a mask built with MODIS and GlobCover data.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- dynamic_ice_flag(num_lines, num_pixels)float32...
- long_name :
- dynamic ice flag
- standard_name :
- status_flag
- source :
- EUMETSAT Ocean and Sea Ice Satellite Applications Facility
- institution :
- EUMETSAT
- flag_meanings :
- no_ice probable_ice ice no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Dynamic ice flag for the location of the KaRIn measurement.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rain_flag(num_lines, num_pixels)float32...
- long_name :
- rain flag
- standard_name :
- status_flag
- flag_meanings :
- no_rain probable_rain rain no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Flag indicates that signal is attenuated, probably from rain.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rad_surface_type_flag(num_lines, num_sides)float32...
- long_name :
- radiometer surface type flag
- standard_name :
- status_flag
- source :
- Advanced Microwave Radiometer
- flag_meanings :
- open_ocean coastal_ocean land
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the validity and type of processing applied to generate the wet troposphere correction (rad_wet_tropo_cor). A value of 0 indicates that open ocean processing is used, a value of 1 indicates coastal processing, and a value of 2 indicates that rad_wet_tropo_cor is invalid due to land contamination.
array([[1., 0.], [1., 0.], [1., 0.], ..., [0., 0.], [0., 0.], [0., 0.]], dtype=float32)
- mean_sea_surface_cnescls(num_lines, num_pixels)float64...
- long_name :
- mean sea surface height (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Mean sea surface height above the reference ellipsoid. The value is referenced to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[34.3678, 34.335 , 34.306 , ..., 32.5267, 32.501 , 32.4758], [34.3202, 34.2876, 34.2562, ..., 32.5841, 32.5518, 32.5209], [34.2707, 34.2378, 34.2054, ..., 32.6479, 32.612 , 32.5783], ..., [30.1714, 30.1627, 30.1533, ..., 30.2082, 30.0953, 29.9772], [30.1742, 30.1672, 30.1616, ..., 30.1103, 30.0095, 29.9012], [30.1834, 30.1789, 30.1758, ..., 30.0096, 29.919 , 29.8205]])
- mean_sea_surface_cnescls_uncert(num_lines, num_pixels)float32...
- long_name :
- mean sea surface height accuracy (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- 0
- valid_max :
- 10000
- comment :
- Accuracy of the mean sea surface height (mean_sea_surface_cnescls).
array([[0.0168, 0.0166, 0.0163, ..., 0.0175, 0.0172, 0.0173], [0.017 , 0.0167, 0.0165, ..., 0.0172, 0.0175, 0.0177], [0.0171, 0.017 , 0.0167, ..., 0.0171, 0.0175, 0.0175], ..., [0.0163, 0.0166, 0.0159, ..., 0.0153, 0.0174, 0.0151], [0.0166, 0.0168, 0.016 , ..., 0.0142, 0.0155, 0.0123], [0.0166, 0.0167, 0.016 , ..., 0.0124, 0.0129, 0.0082]], dtype=float32)
- geoid(num_lines, num_pixels)float64...
- long_name :
- geoid height
- standard_name :
- geoid_height_above_reference_ellipsoid
- source :
- EGM2008 (Pavlis et al., 2012)
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Geoid height above the reference ellipsoid with a correction to refer the value to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[33.4881, 33.45 , 33.4112, ..., 31.8215, 31.8038, 31.7851], [33.4404, 33.4013, 33.3618, ..., 31.8821, 31.8611, 31.8388], [33.3917, 33.3519, 33.3116, ..., 31.9504, 31.9256, 31.899 ], ..., [29.3551, 29.3505, 29.3443, ..., 29.4515, 29.3589, 29.2499], [29.3622, 29.359 , 29.3541, ..., 29.3487, 29.2626, 29.1624], [29.3732, 29.3718, 29.3688, ..., 29.2393, 29.1606, 29.0699]])
- internal_tide_hret(num_lines, num_pixels)float32...
- long_name :
- coherent internal tide (HRET)
- source :
- Zaron (2019)
- units :
- m
- valid_min :
- -2000
- valid_max :
- 2000
- comment :
- Coherent internal ocean tide. This value is subtracted from the ssh_karin and ssh_karin_2 to compute ssha_karin and ssha_karin_2, respectively.
array([[ 0.0038, 0.0042, 0.0046, ..., -0.0021, -0.0017, -0.0013], [ 0.0046, 0.005 , 0.0054, ..., -0.0013, -0.0009, -0.0005], [ 0.0052, 0.0056, 0.006 , ..., -0.0006, -0.0002, 0.0003], ..., [-0.0016, -0.0018, -0.0019, ..., 0.0007, 0.0012, 0.0017], [-0.0014, -0.0015, -0.0017, ..., 0.0009, 0.0014, 0.0018], [-0.0011, -0.0013, -0.0015, ..., 0.0011, 0.0016, 0.002 ]], dtype=float32)
- height_cor_xover(num_lines, num_pixels)float64...
- long_name :
- height correction from crossover calibration
- units :
- m
- quality_flag :
- height_cor_xover_qual
- valid_min :
- -100000
- valid_max :
- 100000
- comment :
- Height correction from crossover calibration. To apply this correction the value of height_cor_xover should be added to the value of ssh_karin, ssh_karin_2, ssha_karin, and ssha_karin_2.
array([[-0.3424, -0.334 , -0.3251, ..., 0.7146, 0.7464, 0.7787], [-0.3425, -0.3341, -0.3252, ..., 0.7147, 0.7465, 0.7788], [-0.3427, -0.3343, -0.3254, ..., 0.7148, 0.7466, 0.7789], ..., [-0.3595, -0.3508, -0.3415, ..., 0.7271, 0.7595, 0.7923], [-0.3597, -0.3509, -0.3416, ..., 0.7272, 0.7596, 0.7924], [-0.3598, -0.3511, -0.3418, ..., 0.7274, 0.7597, 0.7926]])
- height_cor_xover_qual(num_lines, num_pixels)float32...
- long_name :
- quality flag for height correction from crossover calibration
- standard_name :
- status_flag
- flag_meanings :
- good suspect bad
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the quality of the height correction from crossover calibration. Values of 0, 1, and 2 indicate that the correction is good, suspect, and bad, respectively.
array([[2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], ..., [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.]], dtype=float32)
- Conventions :
- CF-1.7
- title :
- Level 2 Low Rate Sea Surface Height Data Product - Basic SSH
- source :
- Ka-band radar interferometer
- history :
- 2023-06-09T22:22:51Z : Creation
- platform :
- SWOT
- reference_document :
- D-56407_SWOT_Product_Description_L2_LR_SSH
- contact :
- podaac@jpl.nasa.gov
- cycle_number :
- 544
- pass_number :
- 17
- short_name :
- L2_LR_SSH
- product_file_id :
- Basic
- crid :
- PIA1
- pge_name :
- PGE_L2_LR_SSH
- pge_version :
- 4.3.0
- time_coverage_start :
- 2023-06-07T00:48:43.660920
- time_coverage_end :
- 2023-06-07T01:34:51.914804
- geospatial_lon_min :
- 93.01029299999999
- geospatial_lon_max :
- 260.464899
- geospatial_lat_min :
- -78.272196
- geospatial_lat_max :
- 78.27232099999999
- left_first_longitude :
- 93.025939
- left_first_latitude :
- -77.053978
- left_last_longitude :
- 260.464899
- left_last_latitude :
- 78.272284
- right_first_longitude :
- 93.01029299999999
- right_first_latitude :
- -78.272148
- right_last_longitude :
- 260.45079599999997
- right_last_latitude :
- 77.054112
- wavelength :
- 0.008385803020979021
- transmit_antenna :
- minus_y
- xref_l1b_lr_intf_file :
- SWOT_L1B_LR_INTF_544_017_20230607T004341_20230607T013455_PIA1_01.nc
- xref_l2_nalt_gdr_files :
- SWOT_IPN_2PfP544_016_20230606_235240_20230607_004345.nc, SWOT_IPN_2PfP544_017_20230607_004345_20230607_013451.nc, SWOT_IPN_2PfP544_018_20230607_013451_20230607_022556.nc
- xref_l2_rad_gdr_files :
- SWOT_IPRAD_2PaP544_016_20230606_235236_20230607_004349_PIA1_01.nc, SWOT_IPRAD_2PaP544_017_20230607_004341_20230607_013455_PIA1_01.nc, SWOT_IPRAD_2PaP544_018_20230607_013447_20230607_022600_PIA1_01.nc
- xref_int_lr_xover_cal_file :
- SWOT_INT_LR_XOverCal_20230606T235230_20230607T234328_PIA1_01.nc
- xref_statickarincal_files :
- SWOT_StaticKaRInCalAdjustableParam_20000101T000000_20991231T235959_20230424T230000_v104.nc
- xref_param_l2_lr_precalssh_file :
- SWOT_Param_L2_LR_PreCalSSH_20000101T000000_20991231T235959_20230215T234922_v201.nc
- xref_orbit_ephemeris_file :
- SWOT_POR_AXVCNE20230608_105153_20230606_225923_20230608_005923.nc
- xref_reforbittrack_files :
- SWOT_RefOrbitTrack125mPass1_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt, SWOT_RefOrbitTrack125mPass2_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt
- xref_meteorological_sealevel_pressure_files :
- SMM_PMA_AXVCNE20230607_050833_20230607_000000_20230607_000000, SMM_PMA_AXVCNE20230607_144811_20230607_060000_20230607_060000
- xref_meteorological_wettroposphere_files :
- SMM_WEA_AXVCNE20230607_050833_20230607_000000_20230607_000000, SMM_WEA_AXVCNE20230607_144811_20230607_060000_20230607_060000
- xref_meteorological_wind_files :
- SMM_VWA_AXVCNE20230607_050833_20230607_000000_20230607_000000, SMM_UWA_AXVCNE20230607_050833_20230607_000000_20230607_000000, SMM_VWA_AXVCNE20230607_144811_20230607_060000_20230607_060000, SMM_UWA_AXVCNE20230607_144811_20230607_060000_20230607_060000
- xref_meteorological_surface_pressure_files :
- SMM_PSA_AXVCNE20230607_054037_20230607_000000_20230607_000000, SMM_PSA_AXVCNE20230607_174035_20230607_060000_20230607_060000
- xref_meteorological_temperature_files :
- SMM_T2M_AXPCNE20230607_054037_20230607_000000_20230607_000000.grb, SMM_T2M_AXPCNE20230607_174035_20230607_060000_20230607_060000.grb
- xref_meteorological_water_vapor_files :
- SMM_CWV_AXPCNE20230607_054037_20230607_000000_20230607_000000.grb, SMM_CWV_AXPCNE20230607_174035_20230607_060000_20230607_060000.grb
- xref_meteorological_cloud_liquid_water_files :
- SMM_CLW_AXPCNE20230607_054037_20230607_000000_20230607_000000.grb, SMM_CLW_AXPCNE20230607_174035_20230607_060000_20230607_060000.grb
- xref_model_significant_wave_height_files :
- SMM_SWH_AXPCNE20230607_054037_20230607_000000_20230607_000000.grb, SMM_SWH_AXPCNE20230607_174035_20230607_060000_20230607_060000.grb
- xref_gim_files :
- JPLQ1580.23I
- xref_pole_location_file :
- SMM_PO1_AXXCNE20230609_201900_19900101_000000_20231207_000000
- xref_dac_files :
- SMM_MOG_AXPCNE20230607_074500_20230607_000000_20230607_000000, SMM_MOG_AXPCNE20230607_204501_20230607_060000_20230607_060000
- xref_precipitation_files :
- SMM_LSR_AXFCNE20230607_065552_20230607_000000_20230607_000000.grb, SMM_CRR_AXFCNE20230607_065552_20230607_000000_20230607_000000.grb, SMM_CRR_AXFCNE20230607_065552_20230607_060000_20230607_060000.grb, SMM_LSR_AXFCNE20230607_065552_20230607_060000_20230607_060000.grb
- xref_sea_ice_mask_files :
- SMM_ICS_AXFCNE20230608_042021_20230607_000000_20230607_235959.nc, SMM_ICN_AXFCNE20230608_041507_20230607_000000_20230607_235959.nc
- xref_wave_model_files :
- SMM_WMA_AXPCNE20230607_072015_20230606_030000_20230607_000000.grb, SMM_WMA_AXPCNE20230608_072015_20230607_030000_20230608_000000.grb
- xref_geco_database_version :
- v100
- ellipsoid_semi_major_axis :
- 6378137.0
- ellipsoid_flattening :
- 0.0033528106647474805
- institution :
- CNES
- references :
- V1.1
- equator_time :
- 2023-06-07T01:09:20.307000Z
- equator_longitude :
- 176.73
- product_version :
- 01
<xarray.Dataset> Dimensions: (num_lines: 112, num_pixels: 69, num_sides: 2) Coordinates: latitude (num_lines, num_pixels) float64 -6... longitude (num_lines, num_pixels) float64 17... Dimensions without coordinates: num_lines, num_pixels, num_sides Data variables: (12/24) time (num_lines) datetime64[ns] 2023-06... time_tai (num_lines) datetime64[ns] 2023-06... ssh_karin (num_lines, num_pixels) float64 na... ssh_karin_qual (num_lines, num_pixels) float64 3.... ssh_karin_uncert (num_lines, num_pixels) float32 na... ssha_karin (num_lines, num_pixels) float64 na... ... ... mean_sea_surface_cnescls (num_lines, num_pixels) float64 34... mean_sea_surface_cnescls_uncert (num_lines, num_pixels) float32 0.... geoid (num_lines, num_pixels) float64 33... internal_tide_hret (num_lines, num_pixels) float32 0.... height_cor_xover (num_lines, num_pixels) float64 -0... height_cor_xover_qual (num_lines, num_pixels) float32 2.... Attributes: (12/60) Conventions: CF-1.7 title: Level 2 Low Rate Sea Surfa... source: Ka-band radar interferometer history: 2023-06-10T22:58:56Z : Cre... platform: SWOT reference_document: D-56407_SWOT_Product_Descr... ... ... ellipsoid_flattening: 0.0033528106647474805 institution: CNES references: V1.1 equator_time: 2023-06-08T00:59:58.158000Z equator_longitude: 176.73 product_version: 01
- num_lines: 112
- num_pixels: 69
- num_sides: 2
- latitude(num_lines, num_pixels)float64-6.413 -6.416 ... -4.605 -4.608
- long_name :
- latitude (positive N, negative S)
- standard_name :
- latitude
- units :
- degrees_north
- valid_min :
- -80000000
- valid_max :
- 80000000
- comment :
- Latitude of measurement [-80,80]. Positive latitude is North latitude, negative latitude is South latitude.
array([[-6.413136, -6.415818, -6.4185 , ..., -6.58883 , -6.591471, -6.594111], [-6.395255, -6.397938, -6.400619, ..., -6.570937, -6.573578, -6.576218], [-6.377375, -6.380057, -6.382738, ..., -6.553044, -6.555685, -6.558325], ..., [-4.463831, -4.466491, -4.469149, ..., -4.638398, -4.641028, -4.643657], [-4.445945, -4.448604, -4.451263, ..., -4.620503, -4.623133, -4.625762], [-4.428059, -4.430718, -4.433376, ..., -4.602608, -4.605238, -4.607867]])
- longitude(num_lines, num_pixels)float64175.2 175.2 175.2 ... 176.7 176.7
- long_name :
- longitude (degrees East)
- standard_name :
- longitude
- units :
- degrees_east
- valid_min :
- 0
- valid_max :
- 359999999
- comment :
- Longitude of measurement. East longitude relative to Greenwich meridian.
array([[175.173285, 175.191164, 175.209043, ..., 176.353686, 176.371577, 176.389468], [175.175968, 175.193847, 175.211725, ..., 176.356329, 176.37422 , 176.392111], [175.178651, 175.196529, 175.214407, ..., 176.358972, 176.376862, 176.394752], ..., [175.46394 , 175.481765, 175.49959 , ..., 176.640654, 176.658488, 176.676321], [175.466591, 175.484416, 175.50224 , ..., 176.643278, 176.661111, 176.678944], [175.469242, 175.487066, 175.50489 , ..., 176.645901, 176.663734, 176.681566]])
- time(num_lines)datetime64[ns]...
- long_name :
- time in UTC
- standard_name :
- time
- tai_utc_difference :
- 37.0
- leap_second :
- 0000-00-00T00:00:00Z
- comment :
- Time of measurement in seconds in the UTC time scale since 1 Jan 2000 00:00:00 UTC. [tai_utc_difference] is the difference between TAI and UTC reference time (seconds) for the first measurement of the data set. If a leap second occurs within the data set, the attribute leap_second is set to the UTC time at which the leap second occurs.
array(['2023-06-08T00:58:05.380654976', '2023-06-08T00:58:05.691124864', '2023-06-08T00:58:06.001444224', '2023-06-08T00:58:06.311719936', '2023-06-08T00:58:06.622104832', '2023-06-08T00:58:06.932304512', '2023-06-08T00:58:07.242725760', '2023-06-08T00:58:07.552944640', '2023-06-08T00:58:07.863292160', '2023-06-08T00:58:08.173624064', '2023-06-08T00:58:08.483852288', '2023-06-08T00:58:08.794282112', '2023-06-08T00:58:09.104456960', '2023-06-08T00:58:09.414602240', '2023-06-08T00:58:09.724722688', '2023-06-08T00:58:10.035069440', '2023-06-08T00:58:10.345412352', '2023-06-08T00:58:10.655624448', '2023-06-08T00:58:10.966043264', '2023-06-08T00:58:11.276240256', '2023-06-08T00:58:11.586611456', '2023-06-08T00:58:11.896905728', '2023-06-08T00:58:12.207162496', '2023-06-08T00:58:12.517560576', '2023-06-08T00:58:12.827748992', '2023-06-08T00:58:13.138127104', '2023-06-08T00:58:13.448342912', '2023-06-08T00:58:13.758676608', '2023-06-08T00:58:14.069008768', '2023-06-08T00:58:14.379230464', '2023-06-08T00:58:14.689642240', '2023-06-08T00:58:14.999830784', '2023-06-08T00:58:15.310213632', '2023-06-08T00:58:15.620479616', '2023-06-08T00:58:15.930755200', '2023-06-08T00:58:16.241134720', '2023-06-08T00:58:16.551314432', '2023-06-08T00:58:16.861672576', '2023-06-08T00:58:17.171862400', '2023-06-08T00:58:17.482219008', '2023-06-08T00:58:17.792524928', '2023-06-08T00:58:18.102763648', '2023-06-08T00:58:18.413165312', '2023-06-08T00:58:18.723349760', '2023-06-08T00:58:19.033746176', '2023-06-08T00:58:19.343988480', '2023-06-08T00:58:19.654277632', '2023-06-08T00:58:19.964645632', '2023-06-08T00:58:20.274801664', '2023-06-08T00:58:20.585058176', '2023-06-08T00:58:20.895209216', '2023-06-08T00:58:21.205592576', '2023-06-08T00:58:21.515864064', '2023-06-08T00:58:21.826125440', '2023-06-08T00:58:22.136509440', '2023-06-08T00:58:22.446693120', '2023-06-08T00:58:22.757099136', '2023-06-08T00:58:23.067318016', '2023-06-08T00:58:23.377631104', '2023-06-08T00:58:23.687969152', '2023-06-08T00:58:23.998138240', '2023-06-08T00:58:24.308426112', '2023-06-08T00:58:24.618587520', '2023-06-08T00:58:24.928976256', '2023-06-08T00:58:25.239219200', '2023-06-08T00:58:25.549500288', '2023-06-08T00:58:25.859860480', '2023-06-08T00:58:26.170046336', '2023-06-08T00:58:26.480455936', '2023-06-08T00:58:26.790653696', '2023-06-08T00:58:27.100988928', '2023-06-08T00:58:27.411299456', '2023-06-08T00:58:27.721483904', '2023-06-08T00:58:28.031779584', '2023-06-08T00:58:28.341942912', '2023-06-08T00:58:28.652338688', '2023-06-08T00:58:28.962557312', '2023-06-08T00:58:29.272857728', '2023-06-08T00:58:29.583194752', '2023-06-08T00:58:29.893387136', '2023-06-08T00:58:30.203794944', '2023-06-08T00:58:30.513977472', '2023-06-08T00:58:30.824334208', '2023-06-08T00:58:31.134617984', '2023-06-08T00:58:31.444813696', '2023-06-08T00:58:31.755095936', '2023-06-08T00:58:32.065265536', '2023-06-08T00:58:32.375666432', '2023-06-08T00:58:32.685862528', '2023-06-08T00:58:32.996179072', '2023-06-08T00:58:33.306489600', '2023-06-08T00:58:33.616691456', '2023-06-08T00:58:33.927088768', '2023-06-08T00:58:34.237259776', '2023-06-08T00:58:34.547624576', '2023-06-08T00:58:34.857895296', '2023-06-08T00:58:35.168029056', '2023-06-08T00:58:35.478186496', '2023-06-08T00:58:35.788371840', '2023-06-08T00:58:36.098777344', '2023-06-08T00:58:36.408951040', '2023-06-08T00:58:36.719301632', '2023-06-08T00:58:37.029576960', '2023-06-08T00:58:37.339799552', '2023-06-08T00:58:37.650178688', '2023-06-08T00:58:37.960342144', '2023-06-08T00:58:38.270724224', '2023-06-08T00:58:38.580947456', '2023-06-08T00:58:38.891182336', '2023-06-08T00:58:39.201440896', '2023-06-08T00:58:39.511635456', '2023-06-08T00:58:39.822032640'], dtype='datetime64[ns]')
- time_tai(num_lines)datetime64[ns]...
- long_name :
- time in TAI
- standard_name :
- time
- tai_utc_difference :
- 37.0
- comment :
- Time of measurement in seconds in the TAI time scale since 1 Jan 2000 00:00:00 TAI. This time scale contains no leap seconds. The difference (in seconds) with time in UTC is given by the attribute [time:tai_utc_difference].
array(['2023-06-08T00:58:42.380654592', '2023-06-08T00:58:42.691124736', '2023-06-08T00:58:43.001443968', '2023-06-08T00:58:43.311719808', '2023-06-08T00:58:43.622104832', '2023-06-08T00:58:43.932305024', '2023-06-08T00:58:44.242725248', '2023-06-08T00:58:44.552944128', '2023-06-08T00:58:44.863292288', '2023-06-08T00:58:45.173624448', '2023-06-08T00:58:45.483851520', '2023-06-08T00:58:45.794282496', '2023-06-08T00:58:46.104456448', '2023-06-08T00:58:46.414602624', '2023-06-08T00:58:46.724722560', '2023-06-08T00:58:47.035069952', '2023-06-08T00:58:47.345411712', '2023-06-08T00:58:47.655624192', '2023-06-08T00:58:47.966043264', '2023-06-08T00:58:48.276240384', '2023-06-08T00:58:48.586611328', '2023-06-08T00:58:48.896905344', '2023-06-08T00:58:49.207161984', '2023-06-08T00:58:49.517560704', '2023-06-08T00:58:49.827748736', '2023-06-08T00:58:50.138126976', '2023-06-08T00:58:50.448342784', '2023-06-08T00:58:50.758675840', '2023-06-08T00:58:51.069008896', '2023-06-08T00:58:51.379231104', '2023-06-08T00:58:51.689641984', '2023-06-08T00:58:51.999831168', '2023-06-08T00:58:52.310214016', '2023-06-08T00:58:52.620478848', '2023-06-08T00:58:52.930755712', '2023-06-08T00:58:53.241134848', '2023-06-08T00:58:53.551315328', '2023-06-08T00:58:53.861673216', '2023-06-08T00:58:54.171862272', '2023-06-08T00:58:54.482218496', '2023-06-08T00:58:54.792524544', '2023-06-08T00:58:55.102763136', '2023-06-08T00:58:55.413165568', '2023-06-08T00:58:55.723350656', '2023-06-08T00:58:56.033746432', '2023-06-08T00:58:56.343988992', '2023-06-08T00:58:56.654277248', '2023-06-08T00:58:56.964646272', '2023-06-08T00:58:57.274801536', '2023-06-08T00:58:57.585057792', '2023-06-08T00:58:57.895209472', '2023-06-08T00:58:58.205592704', '2023-06-08T00:58:58.515863808', '2023-06-08T00:58:58.826126080', '2023-06-08T00:58:59.136509952', '2023-06-08T00:58:59.446692992', '2023-06-08T00:58:59.757098368', '2023-06-08T00:59:00.067317888', '2023-06-08T00:59:00.377631360', '2023-06-08T00:59:00.687968512', '2023-06-08T00:59:00.998137984', '2023-06-08T00:59:01.308425984', '2023-06-08T00:59:01.618587520', '2023-06-08T00:59:01.928976384', '2023-06-08T00:59:02.239220480', '2023-06-08T00:59:02.549499520', '2023-06-08T00:59:02.859860480', '2023-06-08T00:59:03.170046336', '2023-06-08T00:59:03.480455296', '2023-06-08T00:59:03.790653696', '2023-06-08T00:59:04.100989056', '2023-06-08T00:59:04.411299328', '2023-06-08T00:59:04.721483648', '2023-06-08T00:59:05.031779200', '2023-06-08T00:59:05.341943040', '2023-06-08T00:59:05.652338560', '2023-06-08T00:59:05.962558080', '2023-06-08T00:59:06.272857344', '2023-06-08T00:59:06.583194112', '2023-06-08T00:59:06.893387776', '2023-06-08T00:59:07.203795200', '2023-06-08T00:59:07.513977600', '2023-06-08T00:59:07.824333440', '2023-06-08T00:59:08.134617344', '2023-06-08T00:59:08.444813440', '2023-06-08T00:59:08.755095552', '2023-06-08T00:59:09.065265536', '2023-06-08T00:59:09.375666176', '2023-06-08T00:59:09.685862144', '2023-06-08T00:59:09.996179712', '2023-06-08T00:59:10.306489472', '2023-06-08T00:59:10.616691456', '2023-06-08T00:59:10.927088256', '2023-06-08T00:59:11.237259392', '2023-06-08T00:59:11.547624704', '2023-06-08T00:59:11.857895424', '2023-06-08T00:59:12.168028416', '2023-06-08T00:59:12.478185984', '2023-06-08T00:59:12.788371328', '2023-06-08T00:59:13.098776832', '2023-06-08T00:59:13.408951296', '2023-06-08T00:59:13.719301632', '2023-06-08T00:59:14.029576832', '2023-06-08T00:59:14.339798656', '2023-06-08T00:59:14.650177792', '2023-06-08T00:59:14.960341632', '2023-06-08T00:59:15.270723584', '2023-06-08T00:59:15.580947712', '2023-06-08T00:59:15.891182464', '2023-06-08T00:59:16.201441408', '2023-06-08T00:59:16.511634816', '2023-06-08T00:59:16.822032384'], dtype='datetime64[ns]')
- ssh_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using radiometer measurements for wet troposphere effects on the KaRIn measurement (e.g., rad_wet_tropo_cor and sea_state_bias_cor).
array([[ nan, nan, 34.4928, ..., 31.157 , nan, nan], [ nan, nan, 34.4392, ..., 31.2368, nan, nan], [ nan, nan, 34.405 , ..., 31.3316, nan, nan], ..., [ nan, nan, 30.38 , ..., 28.89 , nan, nan], [ nan, nan, 30.3991, ..., 28.7813, nan, nan], [ nan, nan, 30.4081, ..., 28.6864, nan, nan]])
- ssh_karin_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4194811391
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin variable.
array([[3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09]])
- ssh_karin_uncert(num_lines, num_pixels)float32...
- long_name :
- sea surface height anomaly uncertainty
- units :
- m
- valid_min :
- 0
- valid_max :
- 60000
- comment :
- 1-sigma uncertainty on the sea surface height from the KaRIn measurement.
array([[ nan, nan, 0.006 , ..., 0.0072, nan, nan], [ nan, nan, 0.0066, ..., 0.0063, nan, nan], [ nan, nan, 0.0065, ..., 0.0068, nan, nan], ..., [ nan, nan, 0.0078, ..., 0.0075, nan, nan], [ nan, nan, 0.007 , ..., 0.0087, nan, nan], [ nan, nan, 0.0071, ..., 0.0079, nan, nan]], dtype=float32)
- ssha_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.6937, ..., -0.8737, nan, nan], [ nan, nan, 0.6894, ..., -0.8523, nan, nan], [ nan, nan, 0.7056, ..., -0.8223, nan, nan], ..., [ nan, nan, 0.7294, ..., -0.8278, nan, nan], [ nan, nan, 0.7396, ..., -0.8389, nan, nan], [ nan, nan, 0.7338, ..., -0.8334, nan, nan]])
- ssha_karin_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4261920255
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin variable.
array([[3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09]])
- ssh_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_2_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using model-based estimates for wet troposphere effects on the KaRIn measurement (e.g., model_wet_tropo_cor and sea_state_bias_cor_2).
array([[ nan, nan, 34.5602, ..., 31.2213, nan, nan], [ nan, nan, 34.5059, ..., 31.3001, nan, nan], [ nan, nan, 34.4717, ..., 31.3947, nan, nan], ..., [ nan, nan, 30.4451, ..., 28.9492, nan, nan], [ nan, nan, 30.4629, ..., 28.8408, nan, nan], [ nan, nan, 30.4709, ..., 28.7467, nan, nan]])
- ssh_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3792158207
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin_2 variable.
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- ssha_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_2_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin_2 - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.7611, ..., -0.8094, nan, nan], [ nan, nan, 0.7561, ..., -0.789 , nan, nan], [ nan, nan, 0.7723, ..., -0.7592, nan, nan], ..., [ nan, nan, 0.7945, ..., -0.7686, nan, nan], [ nan, nan, 0.8034, ..., -0.7794, nan, nan], [ nan, nan, 0.7966, ..., -0.7731, nan, nan]])
- ssha_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3859267071
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin_2 variable
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- num_pt_avg(num_lines, num_pixels)float32...
- long_name :
- number of samples averaged
- units :
- 1
- valid_min :
- 0
- valid_max :
- 289
- comment :
- Number of native unsmoothed, beam-combined KaRIn samples averaged.
array([[ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], ..., [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.]], dtype=float32)
- distance_to_coast(num_lines, num_pixels)float32...
- long_name :
- distance to coast
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- units :
- m
- valid_min :
- 0
- valid_max :
- 21000
- comment :
- Approximate distance to the nearest coast point along the Earth surface.
array([[130000., 128000., 124000., ..., 30000., 30000., 32000.], [128000., 126000., 124000., ..., 28000., 28000., 30000.], [128000., 126000., 124000., ..., 26000., 26000., 27000.], ..., [147000., 146000., 145000., ..., 126000., 128000., 127000.], [148000., 148000., 147000., ..., 128000., 129000., 128000.], [150000., 149000., 148000., ..., 130000., 131000., 132000.]], dtype=float32)
- heading_to_coast(num_lines, num_pixels)float32...
- long_name :
- heading to coast
- units :
- degrees
- valid_min :
- 0
- valid_max :
- 35999
- comment :
- Approximate compass heading (0-360 degrees with respect to true north) to the nearest coast point.
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], dtype=float32)
- ancillary_surface_classification_flag(num_lines, num_pixels)float32...
- long_name :
- surface classification
- standard_name :
- status_flag
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- flag_meanings :
- open_ocean land continental_water aquatic_vegetation continental_ice_snow floating_ice salted_basin
- flag_values :
- [0 1 2 3 4 5 6]
- valid_min :
- 0
- valid_max :
- 6
- comment :
- 7-state surface type classification computed from a mask built with MODIS and GlobCover data.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- dynamic_ice_flag(num_lines, num_pixels)float32...
- long_name :
- dynamic ice flag
- standard_name :
- status_flag
- source :
- EUMETSAT Ocean and Sea Ice Satellite Applications Facility
- institution :
- EUMETSAT
- flag_meanings :
- no_ice probable_ice ice no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Dynamic ice flag for the location of the KaRIn measurement.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rain_flag(num_lines, num_pixels)float32...
- long_name :
- rain flag
- standard_name :
- status_flag
- flag_meanings :
- no_rain probable_rain rain no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Flag indicates that signal is attenuated, probably from rain.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rad_surface_type_flag(num_lines, num_sides)float32...
- long_name :
- radiometer surface type flag
- standard_name :
- status_flag
- source :
- Advanced Microwave Radiometer
- flag_meanings :
- open_ocean coastal_ocean land
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the validity and type of processing applied to generate the wet troposphere correction (rad_wet_tropo_cor). A value of 0 indicates that open ocean processing is used, a value of 1 indicates coastal processing, and a value of 2 indicates that rad_wet_tropo_cor is invalid due to land contamination.
array([[1., 0.], [1., 0.], [1., 0.], ..., [0., 0.], [0., 0.], [0., 0.]], dtype=float32)
- mean_sea_surface_cnescls(num_lines, num_pixels)float64...
- long_name :
- mean sea surface height (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Mean sea surface height above the reference ellipsoid. The value is referenced to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[34.3678, 34.335 , 34.306 , ..., 32.5267, 32.501 , 32.4758], [34.3202, 34.2876, 34.2562, ..., 32.5841, 32.5518, 32.5209], [34.2707, 34.2378, 34.2054, ..., 32.6479, 32.612 , 32.5783], ..., [30.1714, 30.1627, 30.1533, ..., 30.2082, 30.0953, 29.9772], [30.1742, 30.1672, 30.1616, ..., 30.1103, 30.0095, 29.9012], [30.1834, 30.1789, 30.1758, ..., 30.0096, 29.919 , 29.8205]])
- mean_sea_surface_cnescls_uncert(num_lines, num_pixels)float32...
- long_name :
- mean sea surface height accuracy (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- 0
- valid_max :
- 10000
- comment :
- Accuracy of the mean sea surface height (mean_sea_surface_cnescls).
array([[0.0168, 0.0166, 0.0163, ..., 0.0175, 0.0172, 0.0173], [0.017 , 0.0167, 0.0165, ..., 0.0172, 0.0175, 0.0177], [0.0171, 0.017 , 0.0167, ..., 0.0171, 0.0175, 0.0175], ..., [0.0163, 0.0166, 0.0159, ..., 0.0153, 0.0174, 0.0151], [0.0166, 0.0168, 0.016 , ..., 0.0142, 0.0155, 0.0123], [0.0166, 0.0167, 0.016 , ..., 0.0124, 0.0129, 0.0082]], dtype=float32)
- geoid(num_lines, num_pixels)float64...
- long_name :
- geoid height
- standard_name :
- geoid_height_above_reference_ellipsoid
- source :
- EGM2008 (Pavlis et al., 2012)
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Geoid height above the reference ellipsoid with a correction to refer the value to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[33.4881, 33.45 , 33.4112, ..., 31.8215, 31.8038, 31.7851], [33.4404, 33.4013, 33.3618, ..., 31.8821, 31.8611, 31.8388], [33.3917, 33.3519, 33.3116, ..., 31.9504, 31.9256, 31.899 ], ..., [29.3551, 29.3505, 29.3443, ..., 29.4515, 29.3589, 29.2499], [29.3622, 29.359 , 29.3541, ..., 29.3487, 29.2626, 29.1624], [29.3732, 29.3718, 29.3688, ..., 29.2393, 29.1606, 29.0699]])
- internal_tide_hret(num_lines, num_pixels)float32...
- long_name :
- coherent internal tide (HRET)
- source :
- Zaron (2019)
- units :
- m
- valid_min :
- -2000
- valid_max :
- 2000
- comment :
- Coherent internal ocean tide. This value is subtracted from the ssh_karin and ssh_karin_2 to compute ssha_karin and ssha_karin_2, respectively.
array([[ 0.0004, 0.0007, 0.001 , ..., -0.0021, -0.0013, -0.0006], [ 0.0009, 0.0012, 0.0015, ..., -0.0012, -0.0004, 0.0004], [ 0.0014, 0.0017, 0.002 , ..., -0.0003, 0.0005, 0.0013], ..., [ 0.0019, 0.0018, 0.0018, ..., 0.0013, 0.0016, 0.0018], [ 0.0024, 0.0024, 0.0024, ..., 0.0016, 0.0018, 0.002 ], [ 0.003 , 0.0029, 0.0029, ..., 0.0018, 0.002 , 0.0022]], dtype=float32)
- height_cor_xover(num_lines, num_pixels)float64...
- long_name :
- height correction from crossover calibration
- units :
- m
- quality_flag :
- height_cor_xover_qual
- valid_min :
- -100000
- valid_max :
- 100000
- comment :
- Height correction from crossover calibration. To apply this correction the value of height_cor_xover should be added to the value of ssh_karin, ssh_karin_2, ssha_karin, and ssha_karin_2.
array([[-0.3905, -0.3814, -0.3717, ..., 0.7863, 0.821 , 0.8563], [-0.3906, -0.3815, -0.3718, ..., 0.7863, 0.8211, 0.8564], [-0.3907, -0.3815, -0.3719, ..., 0.7864, 0.8211, 0.8564], ..., [-0.3979, -0.3887, -0.3789, ..., 0.7916, 0.8266, 0.8622], [-0.398 , -0.3887, -0.3789, ..., 0.7916, 0.8266, 0.8622], [-0.398 , -0.3888, -0.379 , ..., 0.7917, 0.8267, 0.8623]])
- height_cor_xover_qual(num_lines, num_pixels)float32...
- long_name :
- quality flag for height correction from crossover calibration
- standard_name :
- status_flag
- flag_meanings :
- good suspect bad
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the quality of the height correction from crossover calibration. Values of 0, 1, and 2 indicate that the correction is good, suspect, and bad, respectively.
array([[2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], ..., [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.]], dtype=float32)
- Conventions :
- CF-1.7
- title :
- Level 2 Low Rate Sea Surface Height Data Product - Basic SSH
- source :
- Ka-band radar interferometer
- history :
- 2023-06-10T22:58:56Z : Creation
- platform :
- SWOT
- reference_document :
- D-56407_SWOT_Product_Description_L2_LR_SSH
- contact :
- podaac@jpl.nasa.gov
- cycle_number :
- 545
- pass_number :
- 17
- short_name :
- L2_LR_SSH
- product_file_id :
- Basic
- crid :
- PIA1
- pge_name :
- PGE_L2_LR_SSH
- pge_version :
- 4.3.0
- time_coverage_start :
- 2023-06-08T00:39:21.524431
- time_coverage_end :
- 2023-06-08T01:25:29.758980
- geospatial_lon_min :
- 93.01029299999999
- geospatial_lon_max :
- 260.464899
- geospatial_lat_min :
- -78.272196
- geospatial_lat_max :
- 78.27232099999999
- left_first_longitude :
- 93.025939
- left_first_latitude :
- -77.053978
- left_last_longitude :
- 260.464899
- left_last_latitude :
- 78.272284
- right_first_longitude :
- 93.01029299999999
- right_first_latitude :
- -78.272148
- right_last_longitude :
- 260.45079599999997
- right_last_latitude :
- 77.054112
- wavelength :
- 0.008385803020979021
- transmit_antenna :
- minus_y
- xref_l1b_lr_intf_file :
- SWOT_L1B_LR_INTF_545_017_20230608T003419_20230608T012533_PIA1_01.nc
- xref_l2_nalt_gdr_files :
- SWOT_IPN_2PfP545_016_20230607_234317_20230608_003423.nc, SWOT_IPN_2PfP545_017_20230608_003423_20230608_012529.nc, SWOT_IPN_2PfP545_018_20230608_012529_20230608_021634.nc
- xref_l2_rad_gdr_files :
- SWOT_IPRAD_2PaP545_016_20230607_234314_20230608_003427_PIA1_01.nc, SWOT_IPRAD_2PaP545_017_20230608_003419_20230608_012532_PIA1_01.nc, SWOT_IPRAD_2PaP545_018_20230608_012525_20230608_021638_PIA1_01.nc
- xref_int_lr_xover_cal_file :
- SWOT_INT_LR_XOverCal_20230607T234307_20230608T233405_PIA1_01.nc
- xref_statickarincal_files :
- SWOT_StaticKaRInCalAdjustableParam_20000101T000000_20991231T235959_20230424T230000_v104.nc
- xref_param_l2_lr_precalssh_file :
- SWOT_Param_L2_LR_PreCalSSH_20000101T000000_20991231T235959_20230215T234922_v201.nc
- xref_orbit_ephemeris_file :
- SWOT_POR_AXVCNE20230609_105508_20230607_225923_20230609_005923.nc
- xref_reforbittrack_files :
- SWOT_RefOrbitTrack125mPass1_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt, SWOT_RefOrbitTrack125mPass2_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt
- xref_meteorological_sealevel_pressure_files :
- SMM_PMA_AXVCNE20230608_050821_20230608_000000_20230608_000000, SMM_PMA_AXVCNE20230608_145143_20230608_060000_20230608_060000
- xref_meteorological_wettroposphere_files :
- SMM_WEA_AXVCNE20230608_050821_20230608_000000_20230608_000000, SMM_WEA_AXVCNE20230608_145143_20230608_060000_20230608_060000
- xref_meteorological_wind_files :
- SMM_VWA_AXVCNE20230608_050821_20230608_000000_20230608_000000, SMM_UWA_AXVCNE20230608_050821_20230608_000000_20230608_000000, SMM_VWA_AXVCNE20230608_145143_20230608_060000_20230608_060000, SMM_UWA_AXVCNE20230608_145143_20230608_060000_20230608_060000
- xref_meteorological_surface_pressure_files :
- SMM_PSA_AXVCNE20230608_054034_20230608_000000_20230608_000000, SMM_PSA_AXVCNE20230608_174034_20230608_060000_20230608_060000
- xref_meteorological_temperature_files :
- SMM_T2M_AXPCNE20230608_054034_20230608_000000_20230608_000000.grb, SMM_T2M_AXPCNE20230608_174034_20230608_060000_20230608_060000.grb
- xref_meteorological_water_vapor_files :
- SMM_CWV_AXPCNE20230608_054034_20230608_000000_20230608_000000.grb, SMM_CWV_AXPCNE20230608_174034_20230608_060000_20230608_060000.grb
- xref_meteorological_cloud_liquid_water_files :
- SMM_CLW_AXPCNE20230608_054034_20230608_000000_20230608_000000.grb, SMM_CLW_AXPCNE20230608_174034_20230608_060000_20230608_060000.grb
- xref_model_significant_wave_height_files :
- SMM_SWH_AXPCNE20230608_054034_20230608_000000_20230608_000000.grb, SMM_SWH_AXPCNE20230608_174034_20230608_060000_20230608_060000.grb
- xref_gim_files :
- JPLQ1590.23I
- xref_pole_location_file :
- SMM_PO1_AXXCNE20230610_200000_19900101_000000_20231208_000000
- xref_dac_files :
- SMM_MOG_AXPCNE20230608_074502_20230608_000000_20230608_000000, SMM_MOG_AXPCNE20230608_204501_20230608_060000_20230608_060000
- xref_precipitation_files :
- SMM_LSR_AXFCNE20230608_065553_20230608_000000_20230608_000000.grb, SMM_CRR_AXFCNE20230608_065553_20230608_000000_20230608_000000.grb, SMM_LSR_AXFCNE20230608_065553_20230608_060000_20230608_060000.grb, SMM_CRR_AXFCNE20230608_065553_20230608_060000_20230608_060000.grb
- xref_sea_ice_mask_files :
- SMM_ICN_AXFCNE20230609_041521_20230608_000000_20230608_235959.nc, SMM_ICS_AXFCNE20230609_042009_20230608_000000_20230608_235959.nc
- xref_wave_model_files :
- SMM_WMA_AXPCNE20230608_072015_20230607_030000_20230608_000000.grb, SMM_WMA_AXPCNE20230609_072011_20230608_030000_20230609_000000.grb
- xref_geco_database_version :
- v100
- ellipsoid_semi_major_axis :
- 6378137.0
- ellipsoid_flattening :
- 0.0033528106647474805
- institution :
- CNES
- references :
- V1.1
- equator_time :
- 2023-06-08T00:59:58.158000Z
- equator_longitude :
- 176.73
- product_version :
- 01
<xarray.Dataset> Dimensions: (num_lines: 112, num_pixels: 69, num_sides: 2) Coordinates: latitude (num_lines, num_pixels) float64 -6... longitude (num_lines, num_pixels) float64 17... Dimensions without coordinates: num_lines, num_pixels, num_sides Data variables: (12/24) time (num_lines) datetime64[ns] 2023-06... time_tai (num_lines) datetime64[ns] 2023-06... ssh_karin (num_lines, num_pixels) float64 na... ssh_karin_qual (num_lines, num_pixels) float64 3.... ssh_karin_uncert (num_lines, num_pixels) float32 na... ssha_karin (num_lines, num_pixels) float64 na... ... ... mean_sea_surface_cnescls (num_lines, num_pixels) float64 34... mean_sea_surface_cnescls_uncert (num_lines, num_pixels) float32 0.... geoid (num_lines, num_pixels) float64 33... internal_tide_hret (num_lines, num_pixels) float32 -0... height_cor_xover (num_lines, num_pixels) float64 -0... height_cor_xover_qual (num_lines, num_pixels) float32 2.... Attributes: (12/60) Conventions: CF-1.7 title: Level 2 Low Rate Sea Surfa... source: Ka-band radar interferometer history: 2023-06-11T22:17:10Z : Cre... platform: SWOT reference_document: D-56407_SWOT_Product_Descr... ... ... ellipsoid_flattening: 0.0033528106647474805 institution: CNES references: V1.1 equator_time: 2023-06-09T00:50:35.975000Z equator_longitude: 176.73 product_version: 01
- num_lines: 112
- num_pixels: 69
- num_sides: 2
- latitude(num_lines, num_pixels)float64-6.413 -6.416 ... -4.605 -4.608
- long_name :
- latitude (positive N, negative S)
- standard_name :
- latitude
- units :
- degrees_north
- valid_min :
- -80000000
- valid_max :
- 80000000
- comment :
- Latitude of measurement [-80,80]. Positive latitude is North latitude, negative latitude is South latitude.
array([[-6.413136, -6.415818, -6.4185 , ..., -6.58883 , -6.591471, -6.594111], [-6.395255, -6.397938, -6.400619, ..., -6.570937, -6.573578, -6.576218], [-6.377375, -6.380057, -6.382738, ..., -6.553044, -6.555685, -6.558325], ..., [-4.463831, -4.466491, -4.469149, ..., -4.638398, -4.641028, -4.643657], [-4.445945, -4.448604, -4.451263, ..., -4.620503, -4.623133, -4.625762], [-4.428059, -4.430718, -4.433376, ..., -4.602608, -4.605238, -4.607867]])
- longitude(num_lines, num_pixels)float64175.2 175.2 175.2 ... 176.7 176.7
- long_name :
- longitude (degrees East)
- standard_name :
- longitude
- units :
- degrees_east
- valid_min :
- 0
- valid_max :
- 359999999
- comment :
- Longitude of measurement. East longitude relative to Greenwich meridian.
array([[175.173285, 175.191164, 175.209043, ..., 176.353686, 176.371577, 176.389468], [175.175968, 175.193847, 175.211725, ..., 176.356329, 176.37422 , 176.392111], [175.178651, 175.196529, 175.214407, ..., 176.358972, 176.376862, 176.394752], ..., [175.46394 , 175.481765, 175.49959 , ..., 176.640654, 176.658488, 176.676321], [175.466591, 175.484416, 175.50224 , ..., 176.643278, 176.661111, 176.678944], [175.469242, 175.487066, 175.50489 , ..., 176.645901, 176.663734, 176.681566]])
- time(num_lines)datetime64[ns]...
- long_name :
- time in UTC
- standard_name :
- time
- tai_utc_difference :
- 37.0
- leap_second :
- 0000-00-00T00:00:00Z
- comment :
- Time of measurement in seconds in the UTC time scale since 1 Jan 2000 00:00:00 UTC. [tai_utc_difference] is the difference between TAI and UTC reference time (seconds) for the first measurement of the data set. If a leap second occurs within the data set, the attribute leap_second is set to the UTC time at which the leap second occurs.
array(['2023-06-09T00:48:43.196505984', '2023-06-09T00:48:43.506753664', '2023-06-09T00:48:43.817081984', '2023-06-09T00:48:44.127431808', '2023-06-09T00:48:44.437660544', '2023-06-09T00:48:44.748079872', '2023-06-09T00:48:45.058283136', '2023-06-09T00:48:45.368800128', '2023-06-09T00:48:45.679163904', '2023-06-09T00:48:45.989424128', '2023-06-09T00:48:46.299820672', '2023-06-09T00:48:46.610016768', '2023-06-09T00:48:46.920433920', '2023-06-09T00:48:47.230658816', '2023-06-09T00:48:47.541003392', '2023-06-09T00:48:47.851330432', '2023-06-09T00:48:48.161569792', '2023-06-09T00:48:48.471977984', '2023-06-09T00:48:48.782177408', '2023-06-09T00:48:49.092650880', '2023-06-09T00:48:49.402947456', '2023-06-09T00:48:49.713233408', '2023-06-09T00:48:50.023605760', '2023-06-09T00:48:50.333807872', '2023-06-09T00:48:50.644225792', '2023-06-09T00:48:50.954429824', '2023-06-09T00:48:51.264790784', '2023-06-09T00:48:51.575090176', '2023-06-09T00:48:51.885341824', '2023-06-09T00:48:52.195733632', '2023-06-09T00:48:52.505927808', '2023-06-09T00:48:52.816374528', '2023-06-09T00:48:53.126625024', '2023-06-09T00:48:53.436935168', '2023-06-09T00:48:53.747280128', '2023-06-09T00:48:54.057491584', '2023-06-09T00:48:54.367906688', '2023-06-09T00:48:54.678098944', '2023-06-09T00:48:54.988477568', '2023-06-09T00:48:55.298747904', '2023-06-09T00:48:55.609022464', '2023-06-09T00:48:55.919392768', '2023-06-09T00:48:56.229597312', '2023-06-09T00:48:56.540093440', '2023-06-09T00:48:56.850332672', '2023-06-09T00:48:57.160657408', '2023-06-09T00:48:57.470980736', '2023-06-09T00:48:57.781203968', '2023-06-09T00:48:58.091607424', '2023-06-09T00:48:58.401791616', '2023-06-09T00:48:58.712181632', '2023-06-09T00:48:59.022430464', '2023-06-09T00:48:59.332719360', '2023-06-09T00:48:59.643080704', '2023-06-09T00:48:59.953265024', '2023-06-09T00:49:00.263638656', '2023-06-09T00:49:00.573822336', '2023-06-09T00:49:00.884181760', '2023-06-09T00:49:01.194467840', '2023-06-09T00:49:01.504715776', '2023-06-09T00:49:01.815099904', '2023-06-09T00:49:02.125279488', '2023-06-09T00:49:02.435679104', '2023-06-09T00:49:02.745899904', '2023-06-09T00:49:03.056212992', '2023-06-09T00:49:03.366541184', '2023-06-09T00:49:03.676754176', '2023-06-09T00:49:03.987179904', '2023-06-09T00:49:04.297364608', '2023-06-09T00:49:04.607734656', '2023-06-09T00:49:04.917995520', '2023-06-09T00:49:05.228257920', '2023-06-09T00:49:05.538626560', '2023-06-09T00:49:05.848806144', '2023-06-09T00:49:06.159209600', '2023-06-09T00:49:06.469411200', '2023-06-09T00:49:06.779738112', '2023-06-09T00:49:07.090052864', '2023-06-09T00:49:07.400245120', '2023-06-09T00:49:07.710578944', '2023-06-09T00:49:08.020745472', '2023-06-09T00:49:08.331133824', '2023-06-09T00:49:08.641363456', '2023-06-09T00:49:08.951652480', '2023-06-09T00:49:09.261994880', '2023-06-09T00:49:09.572181760', '2023-06-09T00:49:09.882585216', '2023-06-09T00:49:10.192767872', '2023-06-09T00:49:10.503119360', '2023-06-09T00:49:10.813390848', '2023-06-09T00:49:11.123650944', '2023-06-09T00:49:11.434068480', '2023-06-09T00:49:11.744242304', '2023-06-09T00:49:12.054632576', '2023-06-09T00:49:12.364845056', '2023-06-09T00:49:12.675150336', '2023-06-09T00:49:12.985470080', '2023-06-09T00:49:13.295668608', '2023-06-09T00:49:13.606062976', '2023-06-09T00:49:13.916233344', '2023-06-09T00:49:14.226600704', '2023-06-09T00:49:14.536844160', '2023-06-09T00:49:14.847117824', '2023-06-09T00:49:15.157495808', '2023-06-09T00:49:15.467666688', '2023-06-09T00:49:15.778062848', '2023-06-09T00:49:16.088252160', '2023-06-09T00:49:16.398578944', '2023-06-09T00:49:16.708876672', '2023-06-09T00:49:17.019089280', '2023-06-09T00:49:17.329477248', '2023-06-09T00:49:17.639641856'], dtype='datetime64[ns]')
- time_tai(num_lines)datetime64[ns]...
- long_name :
- time in TAI
- standard_name :
- time
- tai_utc_difference :
- 37.0
- comment :
- Time of measurement in seconds in the TAI time scale since 1 Jan 2000 00:00:00 TAI. This time scale contains no leap seconds. The difference (in seconds) with time in UTC is given by the attribute [time:tai_utc_difference].
array(['2023-06-09T00:49:20.196505472', '2023-06-09T00:49:20.506753280', '2023-06-09T00:49:20.817081984', '2023-06-09T00:49:21.127432064', '2023-06-09T00:49:21.437660288', '2023-06-09T00:49:21.748079360', '2023-06-09T00:49:22.058282880', '2023-06-09T00:49:22.368801024', '2023-06-09T00:49:22.679164160', '2023-06-09T00:49:22.989423104', '2023-06-09T00:49:23.299820544', '2023-06-09T00:49:23.610016896', '2023-06-09T00:49:23.920433536', '2023-06-09T00:49:24.230658816', '2023-06-09T00:49:24.541003008', '2023-06-09T00:49:24.851330432', '2023-06-09T00:49:25.161570048', '2023-06-09T00:49:25.471977984', '2023-06-09T00:49:25.782177920', '2023-06-09T00:49:26.092650368', '2023-06-09T00:49:26.402948224', '2023-06-09T00:49:26.713234176', '2023-06-09T00:49:27.023605888', '2023-06-09T00:49:27.333807872', '2023-06-09T00:49:27.644226560', '2023-06-09T00:49:27.954430208', '2023-06-09T00:49:28.264790784', '2023-06-09T00:49:28.575090176', '2023-06-09T00:49:28.885341824', '2023-06-09T00:49:29.195733888', '2023-06-09T00:49:29.505928448', '2023-06-09T00:49:29.816373888', '2023-06-09T00:49:30.126624384', '2023-06-09T00:49:30.436935424', '2023-06-09T00:49:30.747279232', '2023-06-09T00:49:31.057491456', '2023-06-09T00:49:31.367906176', '2023-06-09T00:49:31.678098432', '2023-06-09T00:49:31.988477312', '2023-06-09T00:49:32.298747904', '2023-06-09T00:49:32.609022336', '2023-06-09T00:49:32.919392256', '2023-06-09T00:49:33.229597568', '2023-06-09T00:49:33.540093696', '2023-06-09T00:49:33.850333056', '2023-06-09T00:49:34.160657920', '2023-06-09T00:49:34.470980864', '2023-06-09T00:49:34.781203072', '2023-06-09T00:49:35.091607424', '2023-06-09T00:49:35.401791744', '2023-06-09T00:49:35.712181248', '2023-06-09T00:49:36.022430336', '2023-06-09T00:49:36.332719360', '2023-06-09T00:49:36.643080576', '2023-06-09T00:49:36.953264768', '2023-06-09T00:49:37.263638528', '2023-06-09T00:49:37.573822720', '2023-06-09T00:49:37.884182272', '2023-06-09T00:49:38.194467712', '2023-06-09T00:49:38.504715392', '2023-06-09T00:49:38.815100288', '2023-06-09T00:49:39.125279872', '2023-06-09T00:49:39.435679360', '2023-06-09T00:49:39.745899392', '2023-06-09T00:49:40.056212864', '2023-06-09T00:49:40.366541312', '2023-06-09T00:49:40.676753920', '2023-06-09T00:49:40.987179776', '2023-06-09T00:49:41.297364352', '2023-06-09T00:49:41.607733888', '2023-06-09T00:49:41.917995776', '2023-06-09T00:49:42.228258560', '2023-06-09T00:49:42.538627456', '2023-06-09T00:49:42.848806784', '2023-06-09T00:49:43.159209216', '2023-06-09T00:49:43.469410560', '2023-06-09T00:49:43.779737856', '2023-06-09T00:49:44.090052096', '2023-06-09T00:49:44.400245376', '2023-06-09T00:49:44.710578176', '2023-06-09T00:49:45.020745216', '2023-06-09T00:49:45.331133184', '2023-06-09T00:49:45.641363072', '2023-06-09T00:49:45.951651968', '2023-06-09T00:49:46.261995008', '2023-06-09T00:49:46.572181760', '2023-06-09T00:49:46.882586240', '2023-06-09T00:49:47.192768256', '2023-06-09T00:49:47.503119744', '2023-06-09T00:49:47.813390720', '2023-06-09T00:49:48.123650560', '2023-06-09T00:49:48.434068096', '2023-06-09T00:49:48.744241664', '2023-06-09T00:49:49.054632704', '2023-06-09T00:49:49.364845056', '2023-06-09T00:49:49.675150336', '2023-06-09T00:49:49.985470848', '2023-06-09T00:49:50.295668992', '2023-06-09T00:49:50.606063104', '2023-06-09T00:49:50.916233088', '2023-06-09T00:49:51.226600960', '2023-06-09T00:49:51.536844288', '2023-06-09T00:49:51.847116672', '2023-06-09T00:49:52.157495680', '2023-06-09T00:49:52.467667328', '2023-06-09T00:49:52.778063360', '2023-06-09T00:49:53.088252288', '2023-06-09T00:49:53.398579712', '2023-06-09T00:49:53.708876416', '2023-06-09T00:49:54.019089408', '2023-06-09T00:49:54.329476992', '2023-06-09T00:49:54.639642112'], dtype='datetime64[ns]')
- ssh_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using radiometer measurements for wet troposphere effects on the KaRIn measurement (e.g., rad_wet_tropo_cor and sea_state_bias_cor).
array([[ nan, nan, 34.8781, ..., 31.4444, nan, nan], [ nan, nan, 34.8295, ..., 31.5195, nan, nan], [ nan, nan, 34.7802, ..., 31.5787, nan, nan], ..., [ nan, nan, 30.6862, ..., 29.1781, nan, nan], [ nan, nan, 30.7545, ..., 29.0551, nan, nan], [ nan, nan, 30.5205, ..., 28.9572, nan, nan]])
- ssh_karin_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4194811391
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 3.840000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_uncert(num_lines, num_pixels)float32...
- long_name :
- sea surface height anomaly uncertainty
- units :
- m
- valid_min :
- 0
- valid_max :
- 60000
- comment :
- 1-sigma uncertainty on the sea surface height from the KaRIn measurement.
array([[ nan, nan, 0.0073, ..., 0.0073, nan, nan], [ nan, nan, 0.0071, ..., 0.0077, nan, nan], [ nan, nan, 0.0066, ..., 0.0076, nan, nan], ..., [ nan, nan, 0.0132, ..., 0.0095, nan, nan], [ nan, nan, 0.1078, ..., 0.0087, nan, nan], [ nan, nan, 0.1729, ..., 0.0087, nan, nan]], dtype=float32)
- ssha_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.795 , ..., -0.8723, nan, nan], [ nan, nan, 0.7961, ..., -0.8553, nan, nan], [ nan, nan, 0.7976, ..., -0.8607, nan, nan], ..., [ nan, nan, 0.7595, ..., -0.8093, nan, nan], [ nan, nan, 0.8189, ..., -0.8345, nan, nan], [ nan, nan, 0.5701, ..., -0.8319, nan, nan]])
- ssha_karin_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4261920255
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 3.840000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 1.073775e+09, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_2_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using model-based estimates for wet troposphere effects on the KaRIn measurement (e.g., model_wet_tropo_cor and sea_state_bias_cor_2).
array([[ nan, nan, 34.8767, ..., 31.5117, nan, nan], [ nan, nan, 34.8273, ..., 31.5876, nan, nan], [ nan, nan, 34.7775, ..., 31.6464, nan, nan], ..., [ nan, nan, 30.6509, ..., 29.1827, nan, nan], [ nan, nan, 30.8219, ..., 29.0597, nan, nan], [ nan, nan, 30.5884, ..., 28.9618, nan, nan]])
- ssh_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3792158207
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin_2 variable.
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 3.840000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 3.860000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 3.860000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- ssha_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_2_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin_2 - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.7936, ..., -0.805 , nan, nan], [ nan, nan, 0.7939, ..., -0.7872, nan, nan], [ nan, nan, 0.7949, ..., -0.793 , nan, nan], ..., [ nan, nan, 0.7242, ..., -0.8047, nan, nan], [ nan, nan, 0.8863, ..., -0.8299, nan, nan], [ nan, nan, 0.638 , ..., -0.8273, nan, nan]])
- ssha_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3859267071
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin_2 variable
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 3.840000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 3.860000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 3.860000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- num_pt_avg(num_lines, num_pixels)float32...
- long_name :
- number of samples averaged
- units :
- 1
- valid_min :
- 0
- valid_max :
- 289
- comment :
- Number of native unsmoothed, beam-combined KaRIn samples averaged.
array([[ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], ..., [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.]], dtype=float32)
- distance_to_coast(num_lines, num_pixels)float32...
- long_name :
- distance to coast
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- units :
- m
- valid_min :
- 0
- valid_max :
- 21000
- comment :
- Approximate distance to the nearest coast point along the Earth surface.
array([[130000., 128000., 124000., ..., 30000., 30000., 32000.], [128000., 126000., 124000., ..., 28000., 28000., 30000.], [128000., 126000., 124000., ..., 26000., 26000., 27000.], ..., [147000., 146000., 145000., ..., 126000., 128000., 127000.], [148000., 148000., 147000., ..., 128000., 129000., 128000.], [150000., 149000., 148000., ..., 130000., 131000., 132000.]], dtype=float32)
- heading_to_coast(num_lines, num_pixels)float32...
- long_name :
- heading to coast
- units :
- degrees
- valid_min :
- 0
- valid_max :
- 35999
- comment :
- Approximate compass heading (0-360 degrees with respect to true north) to the nearest coast point.
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], dtype=float32)
- ancillary_surface_classification_flag(num_lines, num_pixels)float32...
- long_name :
- surface classification
- standard_name :
- status_flag
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- flag_meanings :
- open_ocean land continental_water aquatic_vegetation continental_ice_snow floating_ice salted_basin
- flag_values :
- [0 1 2 3 4 5 6]
- valid_min :
- 0
- valid_max :
- 6
- comment :
- 7-state surface type classification computed from a mask built with MODIS and GlobCover data.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- dynamic_ice_flag(num_lines, num_pixels)float32...
- long_name :
- dynamic ice flag
- standard_name :
- status_flag
- source :
- EUMETSAT Ocean and Sea Ice Satellite Applications Facility
- institution :
- EUMETSAT
- flag_meanings :
- no_ice probable_ice ice no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Dynamic ice flag for the location of the KaRIn measurement.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rain_flag(num_lines, num_pixels)float32...
- long_name :
- rain flag
- standard_name :
- status_flag
- flag_meanings :
- no_rain probable_rain rain no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Flag indicates that signal is attenuated, probably from rain.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rad_surface_type_flag(num_lines, num_sides)float32...
- long_name :
- radiometer surface type flag
- standard_name :
- status_flag
- source :
- Advanced Microwave Radiometer
- flag_meanings :
- open_ocean coastal_ocean land
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the validity and type of processing applied to generate the wet troposphere correction (rad_wet_tropo_cor). A value of 0 indicates that open ocean processing is used, a value of 1 indicates coastal processing, and a value of 2 indicates that rad_wet_tropo_cor is invalid due to land contamination.
array([[1., 0.], [1., 0.], [1., 0.], ..., [0., 0.], [0., 0.], [0., 0.]], dtype=float32)
- mean_sea_surface_cnescls(num_lines, num_pixels)float64...
- long_name :
- mean sea surface height (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Mean sea surface height above the reference ellipsoid. The value is referenced to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[34.3678, 34.335 , 34.306 , ..., 32.5267, 32.501 , 32.4758], [34.3202, 34.2876, 34.2562, ..., 32.5841, 32.5518, 32.5209], [34.2707, 34.2378, 34.2054, ..., 32.6479, 32.612 , 32.5783], ..., [30.1714, 30.1627, 30.1533, ..., 30.2082, 30.0953, 29.9772], [30.1742, 30.1672, 30.1616, ..., 30.1103, 30.0095, 29.9012], [30.1834, 30.1789, 30.1758, ..., 30.0096, 29.919 , 29.8205]])
- mean_sea_surface_cnescls_uncert(num_lines, num_pixels)float32...
- long_name :
- mean sea surface height accuracy (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- 0
- valid_max :
- 10000
- comment :
- Accuracy of the mean sea surface height (mean_sea_surface_cnescls).
array([[0.0168, 0.0166, 0.0163, ..., 0.0175, 0.0172, 0.0173], [0.017 , 0.0167, 0.0165, ..., 0.0172, 0.0175, 0.0177], [0.0171, 0.017 , 0.0167, ..., 0.0171, 0.0175, 0.0175], ..., [0.0163, 0.0166, 0.0159, ..., 0.0153, 0.0174, 0.0151], [0.0166, 0.0168, 0.016 , ..., 0.0142, 0.0155, 0.0123], [0.0166, 0.0167, 0.016 , ..., 0.0124, 0.0129, 0.0082]], dtype=float32)
- geoid(num_lines, num_pixels)float64...
- long_name :
- geoid height
- standard_name :
- geoid_height_above_reference_ellipsoid
- source :
- EGM2008 (Pavlis et al., 2012)
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Geoid height above the reference ellipsoid with a correction to refer the value to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[33.4881, 33.45 , 33.4112, ..., 31.8215, 31.8038, 31.7851], [33.4404, 33.4013, 33.3618, ..., 31.8821, 31.8611, 31.8388], [33.3917, 33.3519, 33.3116, ..., 31.9504, 31.9256, 31.899 ], ..., [29.3551, 29.3505, 29.3443, ..., 29.4515, 29.3589, 29.2499], [29.3622, 29.359 , 29.3541, ..., 29.3487, 29.2626, 29.1624], [29.3732, 29.3718, 29.3688, ..., 29.2393, 29.1606, 29.0699]])
- internal_tide_hret(num_lines, num_pixels)float32...
- long_name :
- coherent internal tide (HRET)
- source :
- Zaron (2019)
- units :
- m
- valid_min :
- -2000
- valid_max :
- 2000
- comment :
- Coherent internal ocean tide. This value is subtracted from the ssh_karin and ssh_karin_2 to compute ssha_karin and ssha_karin_2, respectively.
array([[-3.700000e-03, -3.600000e-03, -3.400000e-03, ..., -1.800000e-03, -8.000000e-04, 2.000000e-04], [-3.600000e-03, -3.400000e-03, -3.300000e-03, ..., -9.999999e-04, 1.000000e-04, 1.100000e-03], [-3.500000e-03, -3.300000e-03, -3.200000e-03, ..., -1.000000e-04, 9.000000e-04, 2.000000e-03], ..., [ 4.700000e-03, 4.800000e-03, 4.900000e-03, ..., 1.600000e-03, 1.500000e-03, 1.500000e-03], [ 5.400000e-03, 5.500000e-03, 5.600000e-03, ..., 1.800000e-03, 1.700000e-03, 1.600000e-03], [ 6.100000e-03, 6.200000e-03, 6.300000e-03, ..., 2.000000e-03, 1.900000e-03, 1.800000e-03]], dtype=float32)
- height_cor_xover(num_lines, num_pixels)float64...
- long_name :
- height correction from crossover calibration
- units :
- m
- quality_flag :
- height_cor_xover_qual
- valid_min :
- -100000
- valid_max :
- 100000
- comment :
- Height correction from crossover calibration. To apply this correction the value of height_cor_xover should be added to the value of ssh_karin, ssh_karin_2, ssha_karin, and ssha_karin_2.
array([[-0.4378, -0.4251, -0.4121, ..., 0.8156, 0.849 , 0.8828], [-0.4378, -0.4252, -0.4121, ..., 0.8157, 0.8491, 0.8829], [-0.4379, -0.4252, -0.4122, ..., 0.8157, 0.8491, 0.8829], ..., [-0.444 , -0.4311, -0.4179, ..., 0.8189, 0.8523, 0.8862], [-0.444 , -0.4312, -0.4179, ..., 0.8189, 0.8524, 0.8862], [-0.4441, -0.4312, -0.418 , ..., 0.8189, 0.8524, 0.8863]])
- height_cor_xover_qual(num_lines, num_pixels)float32...
- long_name :
- quality flag for height correction from crossover calibration
- standard_name :
- status_flag
- flag_meanings :
- good suspect bad
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the quality of the height correction from crossover calibration. Values of 0, 1, and 2 indicate that the correction is good, suspect, and bad, respectively.
array([[2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], ..., [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.]], dtype=float32)
- Conventions :
- CF-1.7
- title :
- Level 2 Low Rate Sea Surface Height Data Product - Basic SSH
- source :
- Ka-band radar interferometer
- history :
- 2023-06-11T22:17:10Z : Creation
- platform :
- SWOT
- reference_document :
- D-56407_SWOT_Product_Description_L2_LR_SSH
- contact :
- podaac@jpl.nasa.gov
- cycle_number :
- 546
- pass_number :
- 17
- short_name :
- L2_LR_SSH
- product_file_id :
- Basic
- crid :
- PIA1
- pge_name :
- PGE_L2_LR_SSH
- pge_version :
- 4.3.0
- time_coverage_start :
- 2023-06-09T00:29:59.340394
- time_coverage_end :
- 2023-06-09T01:16:07.572978
- geospatial_lon_min :
- 93.01029299999999
- geospatial_lon_max :
- 260.464899
- geospatial_lat_min :
- -78.272196
- geospatial_lat_max :
- 78.27232099999999
- left_first_longitude :
- 93.025939
- left_first_latitude :
- -77.053978
- left_last_longitude :
- 260.464899
- left_last_latitude :
- 78.272284
- right_first_longitude :
- 93.01029299999999
- right_first_latitude :
- -78.272148
- right_last_longitude :
- 260.45079599999997
- right_last_latitude :
- 77.054112
- wavelength :
- 0.008385803020979021
- transmit_antenna :
- minus_y
- xref_l1b_lr_intf_file :
- SWOT_L1B_LR_INTF_546_017_20230609T002457_20230609T011610_PIA1_01.nc
- xref_l2_nalt_gdr_files :
- SWOT_IPN_2PfP546_016_20230608_233355_20230609_002501.nc, SWOT_IPN_2PfP546_017_20230609_002501_20230609_011606.nc, SWOT_IPN_2PfP546_018_20230609_011606_20230609_020712.nc
- xref_l2_rad_gdr_files :
- SWOT_IPRAD_2PaP546_016_20230608_233351_20230609_002504_PIA1_01.nc, SWOT_IPRAD_2PaP546_017_20230609_002457_20230609_011610_PIA1_01.nc, SWOT_IPRAD_2PaP546_018_20230609_011603_20230609_020716_PIA1_01.nc
- xref_int_lr_xover_cal_file :
- SWOT_INT_LR_XOverCal_20230608T233345_20230609T232443_PIA1_01.nc
- xref_statickarincal_files :
- SWOT_StaticKaRInCalAdjustableParam_20000101T000000_20991231T235959_20230424T230000_v104.nc
- xref_param_l2_lr_precalssh_file :
- SWOT_Param_L2_LR_PreCalSSH_20000101T000000_20991231T235959_20230215T234922_v201.nc
- xref_orbit_ephemeris_file :
- SWOT_POR_AXVCNE20230610_105032_20230608_225923_20230610_005923.nc
- xref_reforbittrack_files :
- SWOT_RefOrbitTrack125mPass1_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt, SWOT_RefOrbitTrack125mPass2_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt
- xref_meteorological_sealevel_pressure_files :
- SMM_PMA_AXVCNE20230609_050802_20230609_000000_20230609_000000, SMM_PMA_AXVCNE20230609_145132_20230609_060000_20230609_060000
- xref_meteorological_wettroposphere_files :
- SMM_WEA_AXVCNE20230609_050802_20230609_000000_20230609_000000, SMM_WEA_AXVCNE20230609_145132_20230609_060000_20230609_060000
- xref_meteorological_wind_files :
- SMM_UWA_AXVCNE20230609_050802_20230609_000000_20230609_000000, SMM_VWA_AXVCNE20230609_050802_20230609_000000_20230609_000000, SMM_UWA_AXVCNE20230609_145132_20230609_060000_20230609_060000, SMM_VWA_AXVCNE20230609_145132_20230609_060000_20230609_060000
- xref_meteorological_surface_pressure_files :
- SMM_PSA_AXVCNE20230609_054044_20230609_000000_20230609_000000, SMM_PSA_AXVCNE20230609_174042_20230609_060000_20230609_060000
- xref_meteorological_temperature_files :
- SMM_T2M_AXPCNE20230609_054044_20230609_000000_20230609_000000.grb, SMM_T2M_AXPCNE20230609_174042_20230609_060000_20230609_060000.grb
- xref_meteorological_water_vapor_files :
- SMM_CWV_AXPCNE20230609_054044_20230609_000000_20230609_000000.grb, SMM_CWV_AXPCNE20230609_174042_20230609_060000_20230609_060000.grb
- xref_meteorological_cloud_liquid_water_files :
- SMM_CLW_AXPCNE20230609_054044_20230609_000000_20230609_000000.grb, SMM_CLW_AXPCNE20230609_174042_20230609_060000_20230609_060000.grb
- xref_model_significant_wave_height_files :
- SMM_SWH_AXPCNE20230609_054044_20230609_000000_20230609_000000.grb, SMM_SWH_AXPCNE20230609_174042_20230609_060000_20230609_060000.grb
- xref_gim_files :
- JPLQ1600.23I
- xref_pole_location_file :
- SMM_PO1_AXXCNE20230611_200000_19900101_000000_20231209_000000
- xref_dac_files :
- SMM_MOG_AXPCNE20230609_074502_20230609_000000_20230609_000000, SMM_MOG_AXPCNE20230609_204501_20230609_060000_20230609_060000
- xref_precipitation_files :
- SMM_CRR_AXFCNE20230609_065555_20230609_000000_20230609_000000.grb, SMM_LSR_AXFCNE20230609_065555_20230609_000000_20230609_000000.grb, SMM_LSR_AXFCNE20230609_065555_20230609_060000_20230609_060000.grb, SMM_CRR_AXFCNE20230609_065555_20230609_060000_20230609_060000.grb
- xref_sea_ice_mask_files :
- SMM_ICS_AXFCNE20230610_042009_20230609_000000_20230609_235959.nc, SMM_ICN_AXFCNE20230610_041521_20230609_000000_20230609_235959.nc
- xref_wave_model_files :
- SMM_WMA_AXPCNE20230609_072011_20230608_030000_20230609_000000.grb, SMM_WMA_AXPCNE20230610_072015_20230609_030000_20230610_000000.grb
- xref_geco_database_version :
- v100
- ellipsoid_semi_major_axis :
- 6378137.0
- ellipsoid_flattening :
- 0.0033528106647474805
- institution :
- CNES
- references :
- V1.1
- equator_time :
- 2023-06-09T00:50:35.975000Z
- equator_longitude :
- 176.73
- product_version :
- 01
<xarray.Dataset> Dimensions: (num_lines: 112, num_pixels: 69, num_sides: 2) Coordinates: latitude (num_lines, num_pixels) float64 -6... longitude (num_lines, num_pixels) float64 17... Dimensions without coordinates: num_lines, num_pixels, num_sides Data variables: (12/24) time (num_lines) datetime64[ns] 2023-06... time_tai (num_lines) datetime64[ns] 2023-06... ssh_karin (num_lines, num_pixels) float64 na... ssh_karin_qual (num_lines, num_pixels) float64 3.... ssh_karin_uncert (num_lines, num_pixels) float32 na... ssha_karin (num_lines, num_pixels) float64 na... ... ... mean_sea_surface_cnescls (num_lines, num_pixels) float64 34... mean_sea_surface_cnescls_uncert (num_lines, num_pixels) float32 0.... geoid (num_lines, num_pixels) float64 33... internal_tide_hret (num_lines, num_pixels) float32 -0... height_cor_xover (num_lines, num_pixels) float64 -0... height_cor_xover_qual (num_lines, num_pixels) float32 2.... Attributes: (12/60) Conventions: CF-1.7 title: Level 2 Low Rate Sea Surfa... source: Ka-band radar interferometer history: 2023-06-12T22:33:34Z : Cre... platform: SWOT reference_document: D-56407_SWOT_Product_Descr... ... ... ellipsoid_flattening: 0.0033528106647474805 institution: CNES references: V1.1 equator_time: 2023-06-10T00:41:13.758000Z equator_longitude: 176.73 product_version: 01
- num_lines: 112
- num_pixels: 69
- num_sides: 2
- latitude(num_lines, num_pixels)float64-6.413 -6.416 ... -4.605 -4.608
- long_name :
- latitude (positive N, negative S)
- standard_name :
- latitude
- units :
- degrees_north
- valid_min :
- -80000000
- valid_max :
- 80000000
- comment :
- Latitude of measurement [-80,80]. Positive latitude is North latitude, negative latitude is South latitude.
array([[-6.413136, -6.415818, -6.4185 , ..., -6.58883 , -6.591471, -6.594111], [-6.395255, -6.397938, -6.400619, ..., -6.570937, -6.573578, -6.576218], [-6.377375, -6.380057, -6.382738, ..., -6.553044, -6.555685, -6.558325], ..., [-4.463831, -4.466491, -4.469149, ..., -4.638398, -4.641028, -4.643657], [-4.445945, -4.448604, -4.451263, ..., -4.620503, -4.623133, -4.625762], [-4.428059, -4.430718, -4.433376, ..., -4.602608, -4.605238, -4.607867]])
- longitude(num_lines, num_pixels)float64175.2 175.2 175.2 ... 176.7 176.7
- long_name :
- longitude (degrees East)
- standard_name :
- longitude
- units :
- degrees_east
- valid_min :
- 0
- valid_max :
- 359999999
- comment :
- Longitude of measurement. East longitude relative to Greenwich meridian.
array([[175.173285, 175.191164, 175.209043, ..., 176.353686, 176.371577, 176.389468], [175.175968, 175.193847, 175.211725, ..., 176.356329, 176.37422 , 176.392111], [175.178651, 175.196529, 175.214407, ..., 176.358972, 176.376862, 176.394752], ..., [175.46394 , 175.481765, 175.49959 , ..., 176.640654, 176.658488, 176.676321], [175.466591, 175.484416, 175.50224 , ..., 176.643278, 176.661111, 176.678944], [175.469242, 175.487066, 175.50489 , ..., 176.645901, 176.663734, 176.681566]])
- time(num_lines)datetime64[ns]...
- long_name :
- time in UTC
- standard_name :
- time
- tai_utc_difference :
- 37.0
- leap_second :
- 0000-00-00T00:00:00Z
- comment :
- Time of measurement in seconds in the UTC time scale since 1 Jan 2000 00:00:00 UTC. [tai_utc_difference] is the difference between TAI and UTC reference time (seconds) for the first measurement of the data set. If a leap second occurs within the data set, the attribute leap_second is set to the UTC time at which the leap second occurs.
array(['2023-06-10T00:39:20.978667648', '2023-06-10T00:39:21.288906624', '2023-06-10T00:39:21.599270144', '2023-06-10T00:39:21.909661568', '2023-06-10T00:39:22.219882624', '2023-06-10T00:39:22.530306944', '2023-06-10T00:39:22.840509312', '2023-06-10T00:39:23.150892800', '2023-06-10T00:39:23.461174656', '2023-06-10T00:39:23.771452800', '2023-06-10T00:39:24.081837312', '2023-06-10T00:39:24.392035712', '2023-06-10T00:39:24.702454272', '2023-06-10T00:39:25.012669952', '2023-06-10T00:39:25.323063552', '2023-06-10T00:39:25.633432064', '2023-06-10T00:39:25.943667200', '2023-06-10T00:39:26.254076544', '2023-06-10T00:39:26.564272256', '2023-06-10T00:39:26.874670592', '2023-06-10T00:39:27.184928000', '2023-06-10T00:39:27.495228544', '2023-06-10T00:39:27.805590144', '2023-06-10T00:39:28.115796992', '2023-06-10T00:39:28.426213376', '2023-06-10T00:39:28.736412032', '2023-06-10T00:39:29.046871936', '2023-06-10T00:39:29.357245056', '2023-06-10T00:39:29.667483392', '2023-06-10T00:39:29.977885568', '2023-06-10T00:39:30.288078848', '2023-06-10T00:39:30.598485888', '2023-06-10T00:39:30.908721152', '2023-06-10T00:39:31.219046656', '2023-06-10T00:39:31.529382784', '2023-06-10T00:39:31.839605760', '2023-06-10T00:39:32.150011264', '2023-06-10T00:39:32.460202880', '2023-06-10T00:39:32.770748032', '2023-06-10T00:39:33.081122048', '2023-06-10T00:39:33.391369984', '2023-06-10T00:39:33.701757312', '2023-06-10T00:39:34.011946752', '2023-06-10T00:39:34.322358400', '2023-06-10T00:39:34.632571648', '2023-06-10T00:39:34.942918144', '2023-06-10T00:39:35.253225216', '2023-06-10T00:39:35.563470464', '2023-06-10T00:39:35.873856640', '2023-06-10T00:39:36.184058752', '2023-06-10T00:39:36.494707840', '2023-06-10T00:39:36.805083520', '2023-06-10T00:39:37.115336832', '2023-06-10T00:39:37.425709056', '2023-06-10T00:39:37.735902464', '2023-06-10T00:39:38.046314112', '2023-06-10T00:39:38.356511360', '2023-06-10T00:39:38.666872064', '2023-06-10T00:39:38.977157120', '2023-06-10T00:39:39.287418368', '2023-06-10T00:39:39.597790848', '2023-06-10T00:39:39.907995520', '2023-06-10T00:39:40.218567552', '2023-06-10T00:39:40.528857344', '2023-06-10T00:39:40.839147392', '2023-06-10T00:39:41.149487616', '2023-06-10T00:39:41.459690752', '2023-06-10T00:39:41.770093696', '2023-06-10T00:39:42.080275712', '2023-06-10T00:39:42.390657408', '2023-06-10T00:39:42.700903808', '2023-06-10T00:39:43.011191168', '2023-06-10T00:39:43.321530880', '2023-06-10T00:39:43.631768832', '2023-06-10T00:39:43.942450048', '2023-06-10T00:39:44.252738560', '2023-06-10T00:39:44.563035520', '2023-06-10T00:39:44.873359744', '2023-06-10T00:39:45.183569920', '2023-06-10T00:39:45.493962112', '2023-06-10T00:39:45.804136448', '2023-06-10T00:39:46.114519808', '2023-06-10T00:39:46.424747264', '2023-06-10T00:39:46.735046912', '2023-06-10T00:39:47.045373696', '2023-06-10T00:39:47.355605376', '2023-06-10T00:39:47.666151680', '2023-06-10T00:39:47.976369920', '2023-06-10T00:39:48.286706560', '2023-06-10T00:39:48.596991744', '2023-06-10T00:39:48.907226112', '2023-06-10T00:39:49.217602560', '2023-06-10T00:39:49.527771648', '2023-06-10T00:39:49.838161536', '2023-06-10T00:39:50.148363136', '2023-06-10T00:39:50.458678656', '2023-06-10T00:39:50.768977024', '2023-06-10T00:39:51.079220736', '2023-06-10T00:39:51.389733760', '2023-06-10T00:39:51.699925376', '2023-06-10T00:39:52.010282240', '2023-06-10T00:39:52.320537344', '2023-06-10T00:39:52.630795776', '2023-06-10T00:39:52.941152128', '2023-06-10T00:39:53.251321472', '2023-06-10T00:39:53.561717376', '2023-06-10T00:39:53.871900800', '2023-06-10T00:39:54.182224768', '2023-06-10T00:39:54.492516224', '2023-06-10T00:39:54.802716032', '2023-06-10T00:39:55.113065600', '2023-06-10T00:39:55.423226752'], dtype='datetime64[ns]')
- time_tai(num_lines)datetime64[ns]...
- long_name :
- time in TAI
- standard_name :
- time
- tai_utc_difference :
- 37.0
- comment :
- Time of measurement in seconds in the TAI time scale since 1 Jan 2000 00:00:00 TAI. This time scale contains no leap seconds. The difference (in seconds) with time in UTC is given by the attribute [time:tai_utc_difference].
array(['2023-06-10T00:39:57.978667520', '2023-06-10T00:39:58.288906752', '2023-06-10T00:39:58.599269760', '2023-06-10T00:39:58.909660928', '2023-06-10T00:39:59.219882880', '2023-06-10T00:39:59.530306816', '2023-06-10T00:39:59.840509312', '2023-06-10T00:40:00.150893440', '2023-06-10T00:40:00.461175424', '2023-06-10T00:40:00.771452544', '2023-06-10T00:40:01.081837568', '2023-06-10T00:40:01.392035584', '2023-06-10T00:40:01.702454400', '2023-06-10T00:40:02.012670080', '2023-06-10T00:40:02.323062784', '2023-06-10T00:40:02.633431424', '2023-06-10T00:40:02.943667712', '2023-06-10T00:40:03.254077056', '2023-06-10T00:40:03.564272000', '2023-06-10T00:40:03.874671488', '2023-06-10T00:40:04.184927872', '2023-06-10T00:40:04.495228544', '2023-06-10T00:40:04.805590528', '2023-06-10T00:40:05.115796992', '2023-06-10T00:40:05.426214016', '2023-06-10T00:40:05.736412544', '2023-06-10T00:40:06.046872576', '2023-06-10T00:40:06.357245056', '2023-06-10T00:40:06.667483648', '2023-06-10T00:40:06.977885952', '2023-06-10T00:40:07.288078208', '2023-06-10T00:40:07.598486016', '2023-06-10T00:40:07.908721024', '2023-06-10T00:40:08.219046656', '2023-06-10T00:40:08.529382272', '2023-06-10T00:40:08.839606144', '2023-06-10T00:40:09.150010752', '2023-06-10T00:40:09.460203136', '2023-06-10T00:40:09.770747008', '2023-06-10T00:40:10.081122048', '2023-06-10T00:40:10.391369472', '2023-06-10T00:40:10.701757568', '2023-06-10T00:40:11.011946880', '2023-06-10T00:40:11.322358656', '2023-06-10T00:40:11.632572160', '2023-06-10T00:40:11.942917504', '2023-06-10T00:40:12.253224960', '2023-06-10T00:40:12.563470336', '2023-06-10T00:40:12.873856384', '2023-06-10T00:40:13.184057984', '2023-06-10T00:40:13.494707712', '2023-06-10T00:40:13.805083008', '2023-06-10T00:40:14.115336320', '2023-06-10T00:40:14.425709312', '2023-06-10T00:40:14.735902080', '2023-06-10T00:40:15.046313728', '2023-06-10T00:40:15.356511872', '2023-06-10T00:40:15.666872192', '2023-06-10T00:40:15.977157376', '2023-06-10T00:40:16.287418496', '2023-06-10T00:40:16.597790848', '2023-06-10T00:40:16.907995648', '2023-06-10T00:40:17.218566784', '2023-06-10T00:40:17.528857984', '2023-06-10T00:40:17.839147392', '2023-06-10T00:40:18.149486976', '2023-06-10T00:40:18.459690624', '2023-06-10T00:40:18.770092800', '2023-06-10T00:40:19.080275328', '2023-06-10T00:40:19.390657920', '2023-06-10T00:40:19.700903552', '2023-06-10T00:40:20.011191296', '2023-06-10T00:40:20.321530240', '2023-06-10T00:40:20.631769600', '2023-06-10T00:40:20.942449920', '2023-06-10T00:40:21.252738176', '2023-06-10T00:40:21.563036032', '2023-06-10T00:40:21.873359744', '2023-06-10T00:40:22.183570560', '2023-06-10T00:40:22.493962112', '2023-06-10T00:40:22.804136576', '2023-06-10T00:40:23.114520448', '2023-06-10T00:40:23.424747776', '2023-06-10T00:40:23.735047168', '2023-06-10T00:40:24.045374080', '2023-06-10T00:40:24.355605376', '2023-06-10T00:40:24.666151680', '2023-06-10T00:40:24.976369152', '2023-06-10T00:40:25.286707072', '2023-06-10T00:40:25.596992256', '2023-06-10T00:40:25.907226112', '2023-06-10T00:40:26.217602944', '2023-06-10T00:40:26.527771648', '2023-06-10T00:40:26.838161536', '2023-06-10T00:40:27.148363008', '2023-06-10T00:40:27.458678784', '2023-06-10T00:40:27.768976768', '2023-06-10T00:40:28.079220864', '2023-06-10T00:40:28.389734016', '2023-06-10T00:40:28.699925376', '2023-06-10T00:40:29.010282112', '2023-06-10T00:40:29.320536960', '2023-06-10T00:40:29.630795392', '2023-06-10T00:40:29.941152640', '2023-06-10T00:40:30.251322368', '2023-06-10T00:40:30.561717376', '2023-06-10T00:40:30.871901568', '2023-06-10T00:40:31.182224896', '2023-06-10T00:40:31.492516224', '2023-06-10T00:40:31.802715904', '2023-06-10T00:40:32.113065472', '2023-06-10T00:40:32.423227136'], dtype='datetime64[ns]')
- ssh_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using radiometer measurements for wet troposphere effects on the KaRIn measurement (e.g., rad_wet_tropo_cor and sea_state_bias_cor).
array([[ nan, nan, 35.2186, ..., 31.695 , nan, nan], [ nan, nan, 35.1478, ..., 31.7385, nan, nan], [ nan, nan, 35.1011, ..., 31.8088, nan, nan], ..., [ nan, nan, 31.0551, ..., 29.5036, nan, nan], [ nan, nan, 31.0637, ..., 29.3946, nan, nan], [ nan, nan, 31.0827, ..., 29.2924, nan, nan]])
- ssh_karin_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4194811391
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_uncert(num_lines, num_pixels)float32...
- long_name :
- sea surface height anomaly uncertainty
- units :
- m
- valid_min :
- 0
- valid_max :
- 60000
- comment :
- 1-sigma uncertainty on the sea surface height from the KaRIn measurement.
array([[ nan, nan, 0.0077, ..., 0.0075, nan, nan], [ nan, nan, 0.0072, ..., 0.0081, nan, nan], [ nan, nan, 0.0071, ..., 0.0079, nan, nan], ..., [ nan, nan, 0.0073, ..., 0.0094, nan, nan], [ nan, nan, 0.0087, ..., 0.0083, nan, nan], [ nan, nan, 0.0083, ..., 0.0087, nan, nan]], dtype=float32)
- ssha_karin(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.8231, ..., -0.9348, nan, nan], [ nan, nan, 0.8025, ..., -0.9491, nan, nan], [ nan, nan, 0.8071, ..., -0.943 , nan, nan], ..., [ nan, nan, 0.8175, ..., -0.7904, nan, nan], [ nan, nan, 0.8173, ..., -0.8014, nan, nan], [ nan, nan, 0.8217, ..., -0.8028, nan, nan]])
- ssha_karin_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_ssb_missing bad_radiometer_corr_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 134217728 268435456 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 4261920255
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin variable.
array([[3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 1.073775e+09, 3.758129e+09, 3.758129e+09], ..., [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09], [3.758129e+09, 3.758129e+09, 2.560000e+02, ..., 2.560000e+02, 3.758129e+09, 3.758129e+09]])
- ssh_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height
- standard_name :
- sea surface height above reference ellipsoid
- units :
- m
- quality_flag :
- ssh_karin_2_qual
- valid_min :
- -15000000
- valid_max :
- 150000000
- comment :
- Fully corrected sea surface height measured by KaRIn. The height is relative to the reference ellipsoid defined in the global attributes. This value is computed using model-based estimates for wet troposphere effects on the KaRIn measurement (e.g., model_wet_tropo_cor and sea_state_bias_cor_2).
array([[ nan, nan, 35.222 , ..., 31.7584, nan, nan], [ nan, nan, 35.1506, ..., 31.8031, nan, nan], [ nan, nan, 35.1033, ..., 31.875 , nan, nan], ..., [ nan, nan, 31.1026, ..., 29.5253, nan, nan], [ nan, nan, 31.1108, ..., 29.4178, nan, nan], [ nan, nan, 31.13 , ..., 29.3168, nan, nan]])
- ssh_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- quality flag for sea surface height from KaRIn
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3792158207
- comment :
- Quality flag for sea surface height from KaRIn in ssh_karin_2 variable.
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- ssha_karin_2(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly
- units :
- m
- quality_flag :
- ssha_karin_2_qual
- valid_min :
- -1000000
- valid_max :
- 1000000
- comment :
- Sea surface height anomaly from the KaRIn measurement = ssh_karin_2 - mean_sea_surface_cnescls - solid_earth_tide - ocean_tide_fes – internal_tide_hret - pole_tide - dac.
array([[ nan, nan, 0.8265, ..., -0.8714, nan, nan], [ nan, nan, 0.8053, ..., -0.8845, nan, nan], [ nan, nan, 0.8093, ..., -0.8768, nan, nan], ..., [ nan, nan, 0.865 , ..., -0.7687, nan, nan], [ nan, nan, 0.8644, ..., -0.7782, nan, nan], [ nan, nan, 0.869 , ..., -0.7784, nan, nan]])
- ssha_karin_2_qual(num_lines, num_pixels)float64...
- long_name :
- sea surface height anomaly quality flag
- standard_name :
- status_flag
- flag_meanings :
- suspect_large_ssh_delta suspect_large_ssh_std suspect_large_ssh_window_std suspect_beam_used suspect_less_than_nine_beams suspect_model_swh_used_ssb suspect_ssb_out_of_range suspect_pixel_used suspect_num_pt_avg suspect_orbit_control suspect_sc_event_flag suspect_tvp_qual suspect_volumetric_corr degraded_ssb_not_computable degraded_media_delays_missing degraded_beam_used degraded_large_attitude bad_very_large_attitude bad_tide_corrections_missing bad_outside_of_range degraded bad_not_usable
- flag_masks :
- [ 1 2 4 8 16 32 64 128 256 1024 2048 4096 8192 32768 65536 131072 262144 33554432 67108864 536870912 1073741824 2147483648]
- valid_min :
- 0
- valid_max :
- 3859267071
- comment :
- Quality flag for the SSHA from KaRIn in the ssha_karin_2 variable
array([[2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 4.000000e+02, 2.684355e+09, 2.684355e+09], ..., [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09], [2.684355e+09, 2.684355e+09, 2.560000e+02, ..., 2.560000e+02, 2.684355e+09, 2.684355e+09]])
- num_pt_avg(num_lines, num_pixels)float32...
- long_name :
- number of samples averaged
- units :
- 1
- valid_min :
- 0
- valid_max :
- 289
- comment :
- Number of native unsmoothed, beam-combined KaRIn samples averaged.
array([[ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], [ 0., 0., 136., ..., 119., 0., 0.], ..., [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.], [ 0., 0., 136., ..., 102., 0., 0.]], dtype=float32)
- distance_to_coast(num_lines, num_pixels)float32...
- long_name :
- distance to coast
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- units :
- m
- valid_min :
- 0
- valid_max :
- 21000
- comment :
- Approximate distance to the nearest coast point along the Earth surface.
array([[130000., 128000., 124000., ..., 30000., 30000., 32000.], [128000., 126000., 124000., ..., 28000., 28000., 30000.], [128000., 126000., 124000., ..., 26000., 26000., 27000.], ..., [147000., 146000., 145000., ..., 126000., 128000., 127000.], [148000., 148000., 147000., ..., 128000., 129000., 128000.], [150000., 149000., 148000., ..., 130000., 131000., 132000.]], dtype=float32)
- heading_to_coast(num_lines, num_pixels)float32...
- long_name :
- heading to coast
- units :
- degrees
- valid_min :
- 0
- valid_max :
- 35999
- comment :
- Approximate compass heading (0-360 degrees with respect to true north) to the nearest coast point.
array([[nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], ..., [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan], [nan, nan, nan, ..., nan, nan, nan]], dtype=float32)
- ancillary_surface_classification_flag(num_lines, num_pixels)float32...
- long_name :
- surface classification
- standard_name :
- status_flag
- source :
- MODIS/GlobCover
- institution :
- European Space Agency
- flag_meanings :
- open_ocean land continental_water aquatic_vegetation continental_ice_snow floating_ice salted_basin
- flag_values :
- [0 1 2 3 4 5 6]
- valid_min :
- 0
- valid_max :
- 6
- comment :
- 7-state surface type classification computed from a mask built with MODIS and GlobCover data.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- dynamic_ice_flag(num_lines, num_pixels)float32...
- long_name :
- dynamic ice flag
- standard_name :
- status_flag
- source :
- EUMETSAT Ocean and Sea Ice Satellite Applications Facility
- institution :
- EUMETSAT
- flag_meanings :
- no_ice probable_ice ice no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Dynamic ice flag for the location of the KaRIn measurement.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rain_flag(num_lines, num_pixels)float32...
- long_name :
- rain flag
- standard_name :
- status_flag
- flag_meanings :
- no_rain probable_rain rain no_data
- flag_values :
- [0 1 2 3]
- valid_min :
- 0
- valid_max :
- 3
- comment :
- Flag indicates that signal is attenuated, probably from rain.
array([[0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], ..., [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.], [0., 0., 0., ..., 0., 0., 0.]], dtype=float32)
- rad_surface_type_flag(num_lines, num_sides)float32...
- long_name :
- radiometer surface type flag
- standard_name :
- status_flag
- source :
- Advanced Microwave Radiometer
- flag_meanings :
- open_ocean coastal_ocean land
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the validity and type of processing applied to generate the wet troposphere correction (rad_wet_tropo_cor). A value of 0 indicates that open ocean processing is used, a value of 1 indicates coastal processing, and a value of 2 indicates that rad_wet_tropo_cor is invalid due to land contamination.
array([[1., 0.], [1., 0.], [1., 0.], ..., [0., 0.], [0., 0.], [0., 0.]], dtype=float32)
- mean_sea_surface_cnescls(num_lines, num_pixels)float64...
- long_name :
- mean sea surface height (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Mean sea surface height above the reference ellipsoid. The value is referenced to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[34.3678, 34.335 , 34.306 , ..., 32.5267, 32.501 , 32.4758], [34.3202, 34.2876, 34.2562, ..., 32.5841, 32.5518, 32.5209], [34.2707, 34.2378, 34.2054, ..., 32.6479, 32.612 , 32.5783], ..., [30.1714, 30.1627, 30.1533, ..., 30.2082, 30.0953, 29.9772], [30.1742, 30.1672, 30.1616, ..., 30.1103, 30.0095, 29.9012], [30.1834, 30.1789, 30.1758, ..., 30.0096, 29.919 , 29.8205]])
- mean_sea_surface_cnescls_uncert(num_lines, num_pixels)float32...
- long_name :
- mean sea surface height accuracy (CNES/CLS)
- source :
- CNES_CLS_15
- institution :
- CNES/CLS
- units :
- m
- valid_min :
- 0
- valid_max :
- 10000
- comment :
- Accuracy of the mean sea surface height (mean_sea_surface_cnescls).
array([[0.0168, 0.0166, 0.0163, ..., 0.0175, 0.0172, 0.0173], [0.017 , 0.0167, 0.0165, ..., 0.0172, 0.0175, 0.0177], [0.0171, 0.017 , 0.0167, ..., 0.0171, 0.0175, 0.0175], ..., [0.0163, 0.0166, 0.0159, ..., 0.0153, 0.0174, 0.0151], [0.0166, 0.0168, 0.016 , ..., 0.0142, 0.0155, 0.0123], [0.0166, 0.0167, 0.016 , ..., 0.0124, 0.0129, 0.0082]], dtype=float32)
- geoid(num_lines, num_pixels)float64...
- long_name :
- geoid height
- standard_name :
- geoid_height_above_reference_ellipsoid
- source :
- EGM2008 (Pavlis et al., 2012)
- units :
- m
- valid_min :
- -1500000
- valid_max :
- 1500000
- comment :
- Geoid height above the reference ellipsoid with a correction to refer the value to the mean tide system, i.e. includes the permanent tide (zero frequency).
array([[33.4881, 33.45 , 33.4112, ..., 31.8215, 31.8038, 31.7851], [33.4404, 33.4013, 33.3618, ..., 31.8821, 31.8611, 31.8388], [33.3917, 33.3519, 33.3116, ..., 31.9504, 31.9256, 31.899 ], ..., [29.3551, 29.3505, 29.3443, ..., 29.4515, 29.3589, 29.2499], [29.3622, 29.359 , 29.3541, ..., 29.3487, 29.2626, 29.1624], [29.3732, 29.3718, 29.3688, ..., 29.2393, 29.1606, 29.0699]])
- internal_tide_hret(num_lines, num_pixels)float32...
- long_name :
- coherent internal tide (HRET)
- source :
- Zaron (2019)
- units :
- m
- valid_min :
- -2000
- valid_max :
- 2000
- comment :
- Coherent internal ocean tide. This value is subtracted from the ssh_karin and ssh_karin_2 to compute ssha_karin and ssha_karin_2, respectively.
array([[-0.0077, -0.0077, -0.0077, ..., -0.0012, -0.0002, 0.0008], [-0.008 , -0.008 , -0.008 , ..., -0.0006, 0.0004, 0.0014], [-0.0082, -0.0082, -0.0083, ..., 0. , 0.001 , 0.002 ], ..., [ 0.0061, 0.0063, 0.0066, ..., 0.0015, 0.0012, 0.0008], [ 0.0069, 0.0071, 0.0073, ..., 0.0016, 0.0013, 0.0009], [ 0.0075, 0.0077, 0.0079, ..., 0.0017, 0.0014, 0.001 ]], dtype=float32)
- height_cor_xover(num_lines, num_pixels)float64...
- long_name :
- height correction from crossover calibration
- units :
- m
- quality_flag :
- height_cor_xover_qual
- valid_min :
- -100000
- valid_max :
- 100000
- comment :
- Height correction from crossover calibration. To apply this correction the value of height_cor_xover should be added to the value of ssh_karin, ssh_karin_2, ssha_karin, and ssha_karin_2.
array([[-0.6912, -0.6778, -0.664 , ..., 0.9446, 0.9811, 1.018 ], [-0.6913, -0.6779, -0.6641, ..., 0.9447, 0.9812, 1.0181], [-0.6914, -0.678 , -0.6641, ..., 0.9448, 0.9813, 1.0182], ..., [-0.7015, -0.6878, -0.6736, ..., 0.9561, 0.9929, 1.0301], [-0.7016, -0.6879, -0.6737, ..., 0.9562, 0.993 , 1.0302], [-0.7017, -0.688 , -0.6738, ..., 0.9563, 0.9931, 1.0303]])
- height_cor_xover_qual(num_lines, num_pixels)float32...
- long_name :
- quality flag for height correction from crossover calibration
- standard_name :
- status_flag
- flag_meanings :
- good suspect bad
- flag_values :
- [0 1 2]
- valid_min :
- 0
- valid_max :
- 2
- comment :
- Flag indicating the quality of the height correction from crossover calibration. Values of 0, 1, and 2 indicate that the correction is good, suspect, and bad, respectively.
array([[2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], ..., [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.], [2., 2., 2., ..., 2., 2., 2.]], dtype=float32)
- Conventions :
- CF-1.7
- title :
- Level 2 Low Rate Sea Surface Height Data Product - Basic SSH
- source :
- Ka-band radar interferometer
- history :
- 2023-06-12T22:33:34Z : Creation
- platform :
- SWOT
- reference_document :
- D-56407_SWOT_Product_Description_L2_LR_SSH
- contact :
- podaac@jpl.nasa.gov
- cycle_number :
- 547
- pass_number :
- 17
- short_name :
- L2_LR_SSH
- product_file_id :
- Basic
- crid :
- PIA1
- pge_name :
- PGE_L2_LR_SSH
- pge_version :
- 4.3.0
- time_coverage_start :
- 2023-06-10T00:20:37.128115
- time_coverage_end :
- 2023-06-10T01:06:45.350009
- geospatial_lon_min :
- 93.01029299999999
- geospatial_lon_max :
- 260.464899
- geospatial_lat_min :
- -78.272196
- geospatial_lat_max :
- 78.27232099999999
- left_first_longitude :
- 93.025939
- left_first_latitude :
- -77.053978
- left_last_longitude :
- 260.464899
- left_last_latitude :
- 78.272284
- right_first_longitude :
- 93.01029299999999
- right_first_latitude :
- -78.272148
- right_last_longitude :
- 260.45079599999997
- right_last_latitude :
- 77.054112
- wavelength :
- 0.008385803020979021
- transmit_antenna :
- minus_y
- xref_l1b_lr_intf_file :
- SWOT_L1B_LR_INTF_547_017_20230610T001535_20230610T010648_PIA1_01.nc
- xref_l2_nalt_gdr_files :
- SWOT_IPN_2PfP547_016_20230609_232433_20230610_001538.nc, SWOT_IPN_2PfP547_017_20230610_001538_20230610_010644.nc, SWOT_IPN_2PfP547_018_20230610_010644_20230610_015750.nc
- xref_l2_rad_gdr_files :
- SWOT_IPRAD_2PaP547_016_20230609_232429_20230610_001542_PIA1_01.nc, SWOT_IPRAD_2PaP547_017_20230610_001535_20230610_010648_PIA1_01.nc, SWOT_IPRAD_2PaP547_018_20230610_010640_20230610_015753_PIA1_01.nc
- xref_int_lr_xover_cal_file :
- SWOT_INT_LR_XOverCal_20230609T232423_20230610T231521_PIA1_01.nc
- xref_statickarincal_files :
- SWOT_StaticKaRInCalAdjustableParam_20000101T000000_20991231T235959_20230424T230000_v104.nc
- xref_param_l2_lr_precalssh_file :
- SWOT_Param_L2_LR_PreCalSSH_20000101T000000_20991231T235959_20230215T234922_v201.nc
- xref_orbit_ephemeris_file :
- SWOT_POR_AXVCNE20230611_105049_20230609_225923_20230611_005923.nc
- xref_reforbittrack_files :
- SWOT_RefOrbitTrack125mPass1_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt, SWOT_RefOrbitTrack125mPass2_Cal_20000101T000000_21000101T000000_20200617T193054_v101.txt
- xref_meteorological_sealevel_pressure_files :
- SMM_PMA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_PMA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_wettroposphere_files :
- SMM_WEA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_WEA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_wind_files :
- SMM_UWA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_VWA_AXVCNE20230610_050842_20230610_000000_20230610_000000, SMM_UWA_AXVCNE20230610_144913_20230610_060000_20230610_060000, SMM_VWA_AXVCNE20230610_144913_20230610_060000_20230610_060000
- xref_meteorological_surface_pressure_files :
- SMM_PSA_AXVCNE20230610_054037_20230610_000000_20230610_000000, SMM_PSA_AXVCNE20230610_174038_20230610_060000_20230610_060000
- xref_meteorological_temperature_files :
- SMM_T2M_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_T2M_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_meteorological_water_vapor_files :
- SMM_CWV_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_CWV_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_meteorological_cloud_liquid_water_files :
- SMM_CLW_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_CLW_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_model_significant_wave_height_files :
- SMM_SWH_AXPCNE20230610_054037_20230610_000000_20230610_000000.grb, SMM_SWH_AXPCNE20230610_174038_20230610_060000_20230610_060000.grb
- xref_gim_files :
- JPLQ1610.23I
- xref_pole_location_file :
- SMM_PO1_AXXCNE20230612_200000_19900101_000000_20231210_000000
- xref_dac_files :
- SMM_MOG_AXPCNE20230610_074716_20230610_000000_20230610_000000, SMM_MOG_AXPCNE20230610_204501_20230610_060000_20230610_060000
- xref_precipitation_files :
- SMM_CRR_AXFCNE20230610_065537_20230610_000000_20230610_000000.grb, SMM_LSR_AXFCNE20230610_065537_20230610_000000_20230610_000000.grb, SMM_CRR_AXFCNE20230610_065537_20230610_060000_20230610_060000.grb, SMM_LSR_AXFCNE20230610_065537_20230610_060000_20230610_060000.grb
- xref_sea_ice_mask_files :
- SMM_ICS_AXFCNE20230611_042010_20230610_000000_20230610_235959.nc, SMM_ICN_AXFCNE20230611_041532_20230610_000000_20230610_235959.nc
- xref_wave_model_files :
- SMM_WMA_AXPCNE20230610_072015_20230609_030000_20230610_000000.grb, SMM_WMA_AXPCNE20230611_072014_20230610_030000_20230611_000000.grb
- xref_geco_database_version :
- v100
- ellipsoid_semi_major_axis :
- 6378137.0
- ellipsoid_flattening :
- 0.0033528106647474805
- institution :
- CNES
- references :
- V1.1
- equator_time :
- 2023-06-10T00:41:13.758000Z
- equator_longitude :
- 176.73
- product_version :
- 01
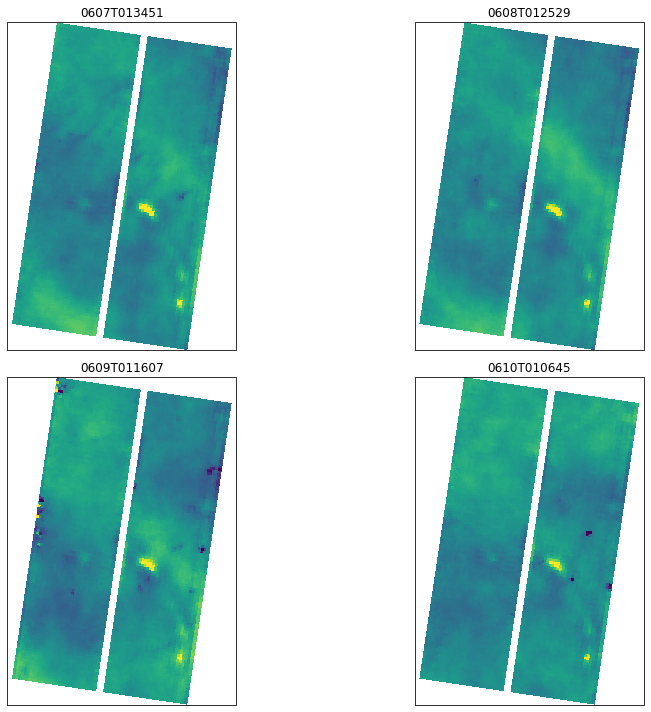
Expert product#
Expert product combines Basic and WindWave products together. The whole workflow is the same as above for the Expert product. I include all code in one block. The plot has ssha_karin_2 and sig0_karin_2.
product_type='Expert'
ss='SWOT_L2_LR_SSH_1.0/'
fns=s3sys.glob(f"s3://podaac-swot-ops-cumulus-protected/SWOT_L2_LR_SSH_1.0/*{product_type}*_{pass_number}_*.nc")
print(f"There are {len(fns)} files in the system. The most recent four files are the following.")
pprint.pprint(fns[-4:])
data=swot.SSH_L2()
data.load_data(fns[-1],lat_bounds=lat_bounds,s3sys=s3sys)
display(getattr(data,product_type))
ni,nj=2,5
fig,ax=plt.subplots(ni,nj,figsize=(14,8),
subplot_kw={'projection': ccrs.PlateCarree()},
sharex=True,sharey=True)
axx=ax.flatten()
data=swot.SSH_L2()
for i in range(nj):
data.load_data(fns[-i-1],lat_bounds=lat_bounds,s3sys=s3sys)
d=getattr(data,product_type)
lon,lat=d['longitude'][:],d['latitude'][:]
#The MSS in the product uses MSS15, which misses a lot of small-scale geoid. Replace it with MSS22 here:
da=d['ssha_karin_2'][:]+d['mean_sea_surface_cnescls']-swot.get_mss22(lon.data, lat.data)-2*d['sea_state_bias_cor_2'].data
da=da-np.nanmean(da,axis=0).reshape(1,-1)
#ax[0,i].scatter(lon,lat,c=da,s=2,vmin=-0.12,vmax=0.12,)
ax[0,i].pcolor(lon,lat,da,vmin=-0.12,vmax=0.12,)
da=d['sig0_karin_2'][:]
da=da-np.nanmean(da,axis=0).reshape(1,-1)
dam=np.nanmedian(da)
dda = np.nanstd(da)
ax[1,i].scatter(lon,lat,c=da,s=2,cmap=plt.cm.binary,vmin=dam-dda*2,vmax=dam+dda*2)
ax[0,i].set_title(fn.split('_')[-3][4:15])
plt.tight_layout()
import cartopy.crs as ccrs
import cartopy.feature as cfeature
import swot_ssh_utils as swot
s3sys=swot.init_S3FileSystem()
product_type='Unsmoothed'
ss='SWOT_L2_LR_SSH_2.0'
fns=s3sys.glob(f"s3://podaac-swot-ops-cumulus-protected/{ss}/*{product_type}*_{pass_number}_*.nc")
dd=swot.SSH_L2()
print(len(fns),fns[-1])
product_type='Expert'
fns=s3sys.glob(f"s3://podaac-swot-ops-cumulus-protected/{ss}/*{product_type}*_{pass_number}_*.nc")
print(f"There are {len(fns)} files in the system. The most recent four files are the following.")
pprint.pprint(fns[-4:])
data=swot.SSH_L2()
data.load_data(fns[-1],lat_bounds=lat_bounds,s3sys=s3sys)
display(getattr(data,product_type))
fig, ax = plt.subplots(figsize=(8, 8), subplot_kw=dict(projection=ccrs.PlateCarree()))
land = cfeature.NaturalEarthFeature('physical', 'land', '10m', edgecolor='face', facecolor='gray')
#bathymetry = cfeature.NaturalEarthFeature('physical', 'bathymetry', '110m', edgecolor='face', facecolor=cfeature.COLORS['water'])
ax.add_feature(land)
data=swot.SSH_L2()
data.load_data(fns[-2],lat_bounds=lat_bounds,s3sys=s3sys)
d=getattr(data,product_type)
lon,lat=d['longitude'][:],d['latitude'][:]
#The MSS in the product uses MSS15, which misses a lot of small-scale geoid. Replace it with MSS22 here:
da=d['ssha_karin_2'][:]+d['height_cor_xover'][:]
da=da-np.nanmean(da,axis=0).reshape(1,-1)
#display(d)
#da=np.where(d['ssha_karin_2_qual']==0,da,np.nan)
lon_out,lat_out=np.arange(175.5,176.5,0.01),np.arange(-6.5,-5.2,0.01)
d_out=swot.resample_swath_data(lon, lat, ssh, lon_out, lat_out, sigma=1000, radius_of_influence = 1000)
im=ax.pcolor(lon,lat,da,vmin=-0.12,vmax=0.12,cmap=plt.cm.Spectral_r,facecolor='gray')
# Add latitude and longitude labels
ax.set_xticks(np.arange(175,177, 0.5)) # Adjust the interval as needed
ax.set_yticks(np.arange(-7,-4, 0.5)) # Adjust the interval as needed
ax.set_xticklabels(ax.get_xticks(), rotation=45)
ax.set_yticklabels(ax.get_yticks())
# Add a colorbar
cbar = plt.colorbar(im, ax=ax)
cbar.set_label('SSH')
plt.xlim(175.5,176.5)
plt.ylim(-6.5,-5.2)